You want to learn to program? Learning computer programming can be tricky, and you may be thinking about taking a certain course. This may be true for some programming languages, but there are many that only take a day or two to understand the basics. Python is one of those languages. You can run basic Python programs in just a few minutes. See Step 1 below to find out how.
Step
Part 1 of 5: Installing Python (Windows)
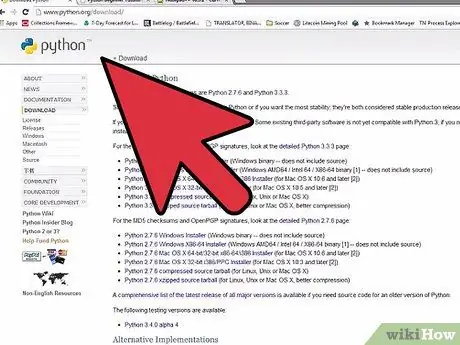
Step 1. Download Python for Windows system
The Windows Python interpreter can be downloaded for free from the Python site. Make sure to download the correct version for your operating system.
- You will need to download the latest version available, which is 3.4 at the time of this writing.
- OS X and Linux come with Python. You may not need to install any Python related software, but you can install a text editor.
- Most versions of Linux distributions and OS X still use Python 2.x. There are some minor differences between versions 2 & 3, but the most significant change is in the "print" statement. To install the latest version of Python on OS X or Linux, you can download the file from the Python website.
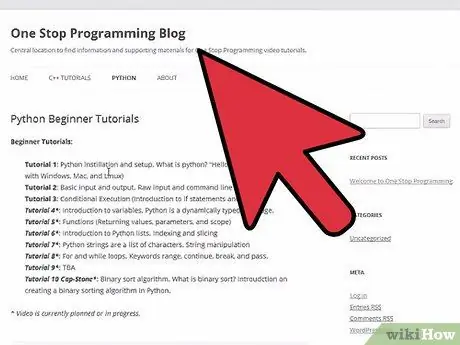
Step 2. Install the Python interpreter
Most users can install the interpreter without changing any settings. You can integrate Python into the Command Prompt by enabling the last option in the list of available modules.
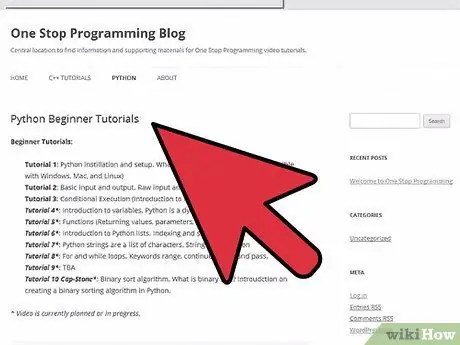
Step 3. Install a text editor
While you can create Python programs from Notepad or TextEdit, it's much easier to read and write code using a dedicated text editor. There are various free editors you can use, such as Notepad++ (Windows), TextWrangler (Mac), or jEdit (Any system).
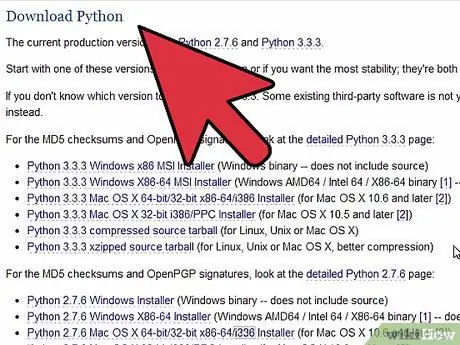
Step 4. Test the installation
Open Command Prompt (Windows) from Terminal (Mac/Linux) and type python. Python will be loaded and the version number will be displayed. You will be taken to the Python interpreter command prompt, displayed as >>>.
Type print("Hello, World!") and press Enter. You will see the text displayed below the Python command line
Part 2 of 5: Learning the Basic Concepts
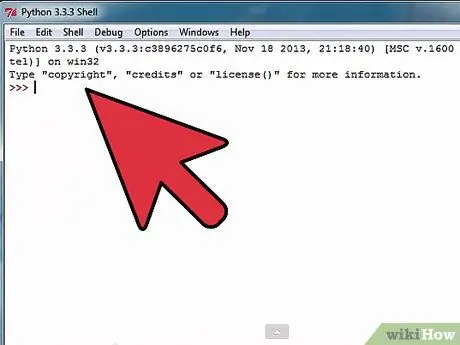
Step 1. Understand that Python does not need to be compiled
Python is an interpreted language, meaning you can run programs as soon as you make changes to files. This makes the process of iterating, revising, and troubleshooting programs much faster than in many other languages.
Python is one of the easier languages to learn, and you can run basic programs in just a few minutes
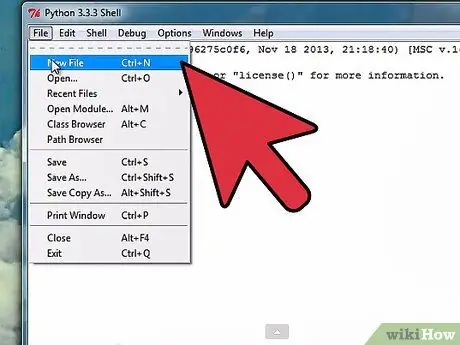
Step 2. Fiddle with the interpreter
You can use the interpreter to test code without first adding it to the program. This is great for learning how special commands work, or writing throwaway programs.
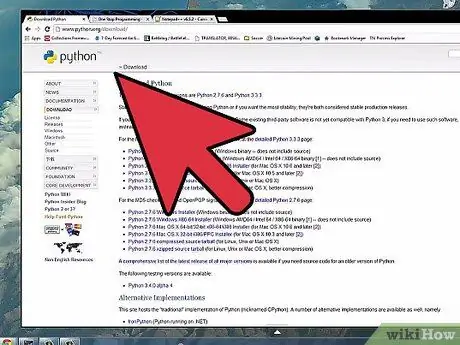
Step 3. Learn how Python handles objects and variables
Python is an object-oriented language, which means that everything in the program is treated as an object. This means that you don't have to declare variables at the beginning of the program (you can do this at any time), and you don't have to specify the type of the variable (integer, string, etc).
Part 3 of 5: Using the Python Interpreter As a Calculator
Performing some basic calculator functions will help you become familiar with Python syntax and how to handle numbers and strings.
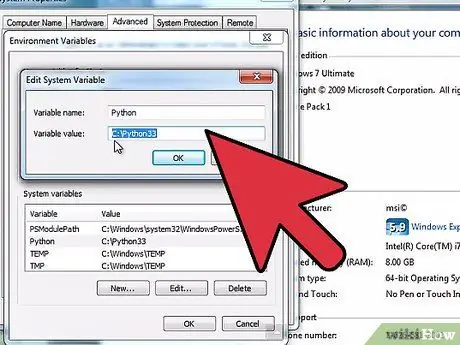
Step 1. Run the interpreter
Open Command Prompt or Terminal. Type python at the prompt and press Enter. This will load the Python interpreter and you will be taken to the Python command prompt (>>>).
If you don't integrate Python in the command prompt, you'll need to navigate to the Python folder to run the interpreter
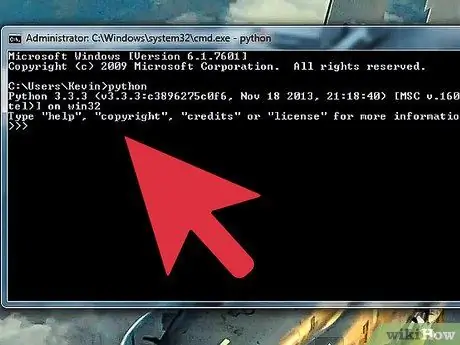
Step 2. Perform basic arithmetic
You can use Python to do basic arithmetic easily. See the box below for some examples of how to use the calculator functions. Note: # are comments in Python code, and they are not processed by the interpreter.
>> 3 + 7 10 >>> 100 - 10*3 70 >>> (100 - 10*3) / 2 # Division will always return a floating point number (decimal) 35.0 >>> (100 - 10*3) // 2 # Rounding down division (two slashes) will throw away the decimal 35 >>> 23 % 4 # This will calculate the remainder of division 3 >>> 17.53 * 2.67 / 4.1 11.41587804878049
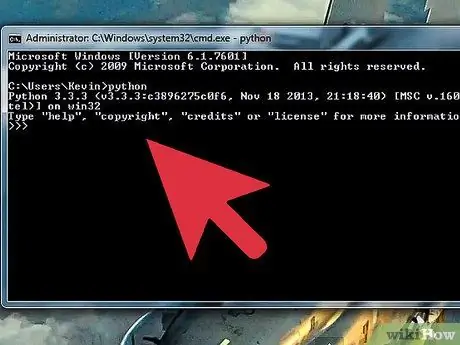
Step 3. Calculate the rank
You can use the ** operator to denote exponents. Python can quickly calculate large powers. See the box below for an example.
>> 7 ** 2 # 7 squared 49 >>> 5 ** 7 # 5 to the power of 7 78125
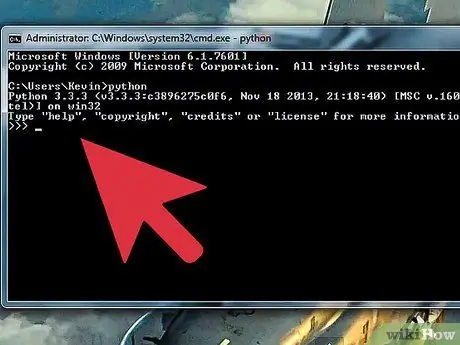
Step 4. Create and manipulate variables
You can assign variables in Python to do basic algebra. This is a great introduction to knowing how to assign variables in Python programs. Variables are specified using the = sign. See the box below for an example.
>> a = 5 >>> b = 4 >>> a * b 20 >>> 20 * a // b 25 >>> b ** 2 16 >>> width = 10 # Variable can be any string >>> height = 5 >>> width * height 50
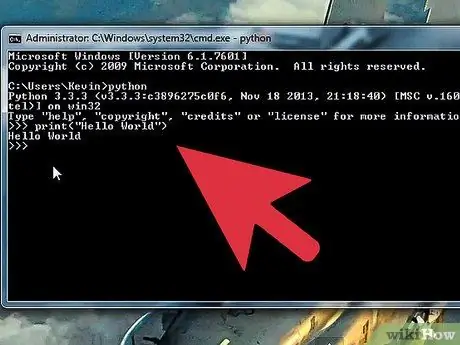
Step 5. Close the interpreter
When you're done using the interpreter, you can close it and return to the command prompt by pressing Ctrl+Z (Windows) or Ctrl+D (Linux/Mac) and then pressing Enter. You can also type quit() and press Enter.
Part 4 of 5: Creating the First Program
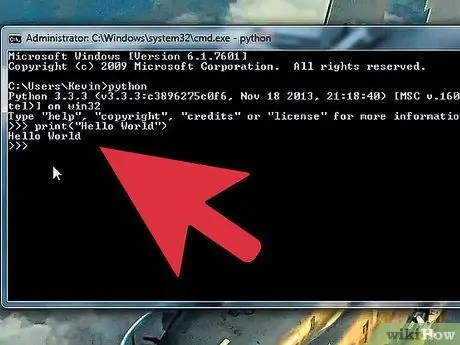
Step 1. Open a text editor
You can by creating a test program that will familiarize you with the basics of creating and saving programs, then running them through the interpreter. This will also help you test that the interpreter is installed correctly.
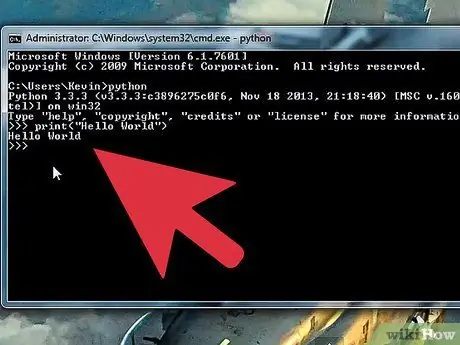
Step 2. Create a "print" statement
"Print" is one of the basic Python functions used to display information in the terminal during a program. Note: "print" is one of the biggest changes from Python 2 to Python 3. In Python 2, you only need to type "print" followed by what you want to display. In Python 3, "print" has become a function, so you have to type "print()", and write what you want in parentheses.
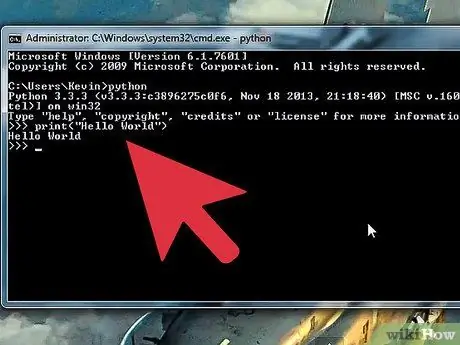
Step 3. Add a statement
One of the most common ways to test a programming language is to display the text "Hello, World!" Enclose this piece of text in the "print()" statement, including the quotes:
print("Hello, World!")
Unlike most other languages, you don't need to declare line endings with;. You also don't need to use curly braces ({}) to represent blocks. Instead, the indent will indicate what is included in the block
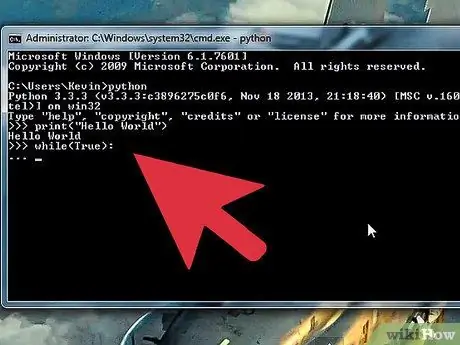
Step 4. Save the file
Click the File menu in the text editor and select Save As. In the drop-down menu under the name box, select the Python file type. If you're using Notepad (but not recommended), select "All Files" and then add "py" to the end of the filename.
- Make sure to save the file somewhere easy to access, as you'll have to point it at the command prompt.
- For this example, save the file as "hello.py".
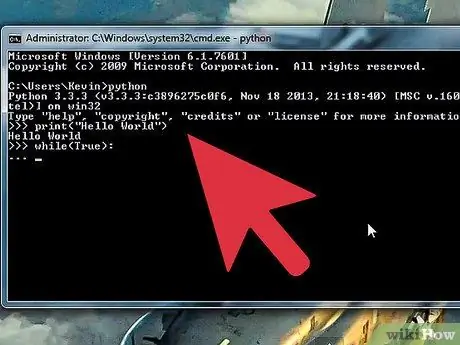
Step 5. Run the program
Open Command Prompt or Terminal and navigate to the location where you saved the file. Once there, run the file by typing hello.py and Enter. You will see the text displayed below the command prompt.
Depending on how you installed Python, you may need to type python hello.py to run the program
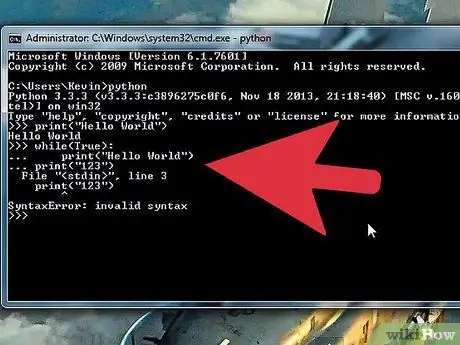
Step 6. Test the program frequently
One of the great things about Python is that you can test new programs right away. Another advantage is that your command prompt and editor are open. After saving changes in the editor, you can run the program directly from the command line, making it quick to test changes.
Part 5 of 5: Building Advanced Programs
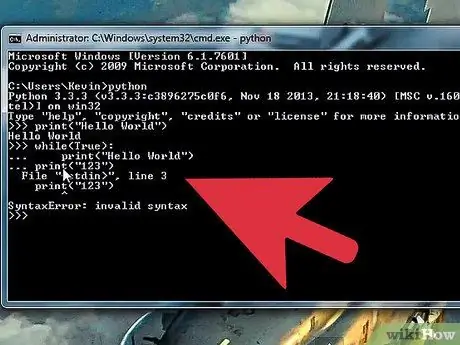
Step 1. Experiment with basic flow control statements
Flow control statements are useful for controlling what a program does under certain conditions. These statements are the core of Python programming, so you can create programs that do a variety of things, depending on input and conditions. The while statement is a good start to learn. In this example, you use a while statement to calculate the Fibonacci series up to 100:
# Each number in the Fibonacci sequence is # the sum of the previous two numbers a, b = 0, 1 while b < 100: print(b, end=' ') a, b = b, a+b
- The sequence will run as long as (while) b is less than (<) 100.
- Program outputs are 1 1 2 3 5 8 13 21 34 55 89
- The command end=' ' will output the output on the same line instead of putting each value on a separate line.
-
There are a few things to note in this simple program, and they are very important for creating complex programs in Python:
- Pay attention to the indents.: indicates that the next line will be indented and is part of the block. In the example above, print(b) and a, b = b, a+b are part of the while block. Correct indentation is essential for the program to work.
- Multiple variables can be defined on the same line. In the example above, a and b. Both are defined on the first line
- If you enter the program directly into the interpreter, you must add a blank line at the end so that the interpreter knows that the program has finished.
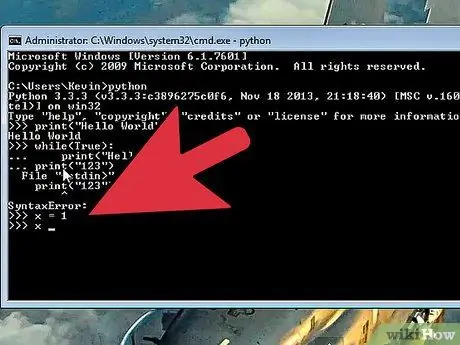
Step 2. Build the function in the program
You can define functions that can later be called in the program. This is especially useful if you need to use multiple functions within the confines of a larger program. In the following example, you can create a function to call a Fibonacci sequence similar to the one you wrote earlier:
def fib(n): a, b = 0, 1 while a < n: print(a, end=' ') a, b = b, a+b print() # You can then call the # Fibonacci function for each value specified fib(1000)
This will return 0 1 1 2 3 5 8 13 21 34 55 89 144 233 377 610 987
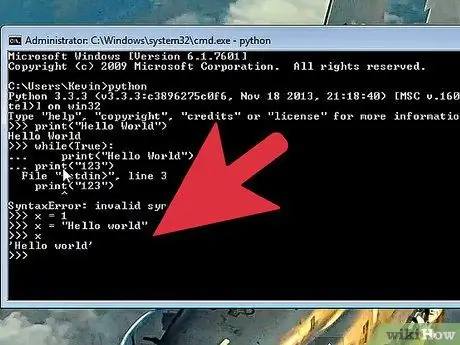
Step 3. Build a more complex flow control program
Flow control statements are useful for setting certain conditions that change how the program is executed. This is especially important if you are dealing with user input. The following example will use if, elif (else if), and else to create a simple program that evaluates a user's age.
age = int(input("Enter your age: ")) if age <= 12: print("Childhood is amazing!") elif age in range(13, 20): print("You are a teenager!") else: print("Time to grow up") # If any of these statements are true # The corresponding message will be displayed. # If none of the statements are true, # an "else" message will be displayed.
-
The program also introduces some other very important statements for use in various applications:
- input() - This calls user input from the keyboard. The user will see the message written in brackets. In this example, input() is surrounded by the int() function, which means all inputs will be treated as integers
- range() - This function can be used in a variety of ways. In this program this function checks if a number is in the range 13 and 20. The end of the range is not counted in the calculation.
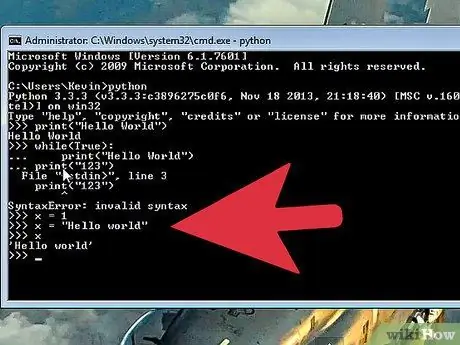
Step 4. Learn other conditional expressions
The previous example uses the "less than or equal to" symbol (<=) to determine whether the entered age matches the condition. You can use the same conditional expressions as in math, but the way they are typed is slightly different:
Meaning | Symbol | Python Symbol | |
---|---|---|---|
Smaller than | < | < | |
Greater than | > | > | |
Less than or equal to | ≤ | <= | |
Greater than or equal to | ≥ | >= | |
Together with | = | == | |
Not equal to | ≠ | != |
Step 5. Constantly learning
All of the above are just Python basics. While Python is one of the simplest languages to learn, there's a lot of scope in it that you can dig into. The best way to keep learning is to keep programming! Remember that you can quickly write a program from scratch directly from the interpreter, and testing your changes is as easy as running the program again from the command line.
- There are many good books available on Python programming, including "Python for Beginners", "Python Cookbook", and "Python Programming: An Introduction to Computer Science".
- There are various sources available on the internet, but most are still devoted to Python 2.x. You will have to make adjustments for each given example.
- Many courses offer Python learning. Python is often taught in introductory classes, because it is one of the easier languages to learn.