Flash is a popular format for browser video games, such as sites like Newgrounds and Kongregate. Although the Flash format tends to be underutilized in mobile applications, there are still many quality games that continue to be made using Flash. Flash uses ActionScript, a language that is easy to learn and provides control over objects on the screen. See Step 1 below to learn how to create a basic Flash game.
Step
Part 1 of 3: Getting Started
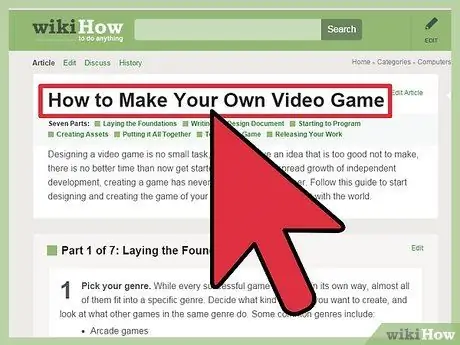
Step 1. Design the game
Before you start coding, create a rough idea of your game. Flash is best suited for simple games, so focus on creating games that have very few gameplay mechanics. Define the genre and game mechanics before starting the prototype. Common flash games include:
- Endless runner: The game automatically moves characters. Players have to jump over obstacles or interact with the game. Players usually only have one or two control options.
- Fighters: The game is usually side-scrolling. Players must defeat the enemy in order to advance. The player character has several moves to defeat the enemy.
- Puzzles: Players must solve puzzles to beat each level. Starting from the style of bringing together three-of-a-kind objects like Bejeweled, to complex puzzles like adventure games.
- RPG: The game focuses on character development and progress. Players move through many different situations as well as different types of enemies. The combat mechanics vary widely, but many of these types are turn-based. RPGs are significantly more difficult to code than simple action games.
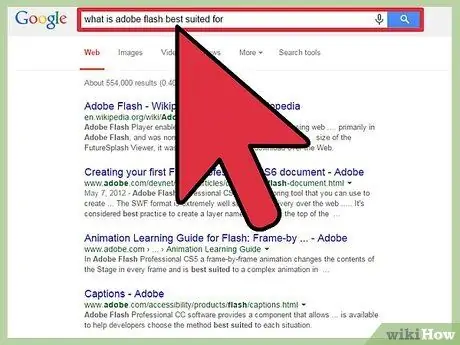
Step 2. Understand what the advantages of flash are
Flash is most suitable for 2D games. Flash can be made to create 3D games, but it is very complicated and requires more knowledge. Almost every successful Flash game has a 2D format.
Flash games are also best suited for quick sessions. This is because most players play when they have little free time, such as downtime, which means gaming sessions are usually 15 minutes long at most
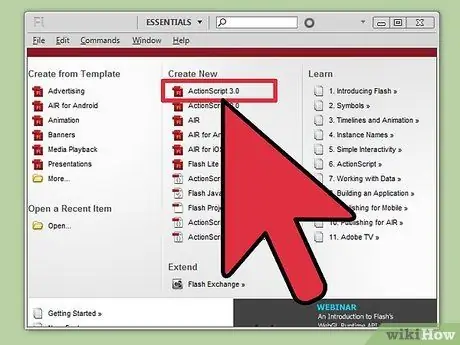
Step 3. Familiarize yourself with the ActionScript3 (AS3) language
Flash games are programmed in AS3, and you will need to have some basic understanding of how they work to be successful at creating games. You can create simple games with a basic understanding of code in AS3.
There are many ActionScript books available on Amazon and other stores, along with a variety of tutorials and happenings on the internet
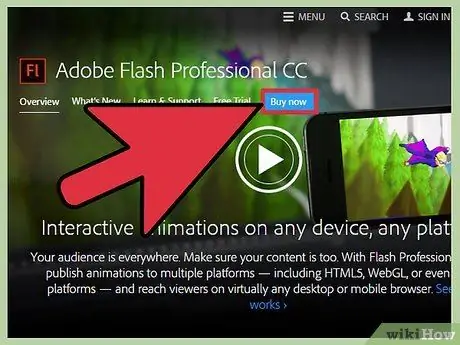
Step 4. Download Flash Professional
This program is a little pricey, but it is very good for creating flash programs quickly. There are several other program options, including open source ones, but they are generally less compatible or take longer to complete the same task.
Flash Professional is the only program you'll need to start creating games
Part 2 of 3: Writing Basic Games
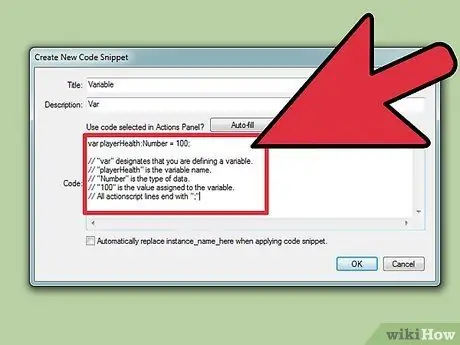
Step 1. Understand the basic building blocks of AS3 code
The base game has several different code structures. There are three main parts to AS3 code:
-
Variables - This is about how data is stored. Data can be numbers, words (strings), objects, and more. Variables are defined by the var code and must consist of one word.
var playerHealth:Number = 100; // "var" indicates that you are defining a variable. // "playerHealth" is the variable name. // "Number" is the data type. // "100" is the value assigned to the variable. // All actionscript lines end with ";"
-
Event handler - event handler looks for certain things that happened, then notifies the rest of the program. This is important for player input and repeated code. Event handlers usually call functions.
addEventListener(MouseEvent. CLICK, swingSword); // "addEventListener()" defines an event handler. // "MouseEvent" is the category of input being listened to. // ". CLICK" Is a specified event in the MouseEvent category. // "swingSword" is the function that is called when the event occurs.
-
Function - A piece of code that is assigned to a keyword and can be called later. Functions handle most game programming, and a complex game can have hundreds of functions. Functions can be in any order because they only work when they are called.
function swingSword(e:MouseEvent):void; { //Your code here } // "function" is the keyword that appears at the beginning of every function. // "swingSword" is the name of the function. // "e:MouseEvent" is an added parameter, indicating // that the function was called from the event listener. // ":void" is the value returned by the function. // If no value is returned, use:void.
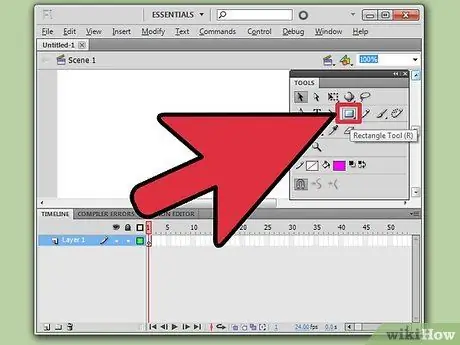
Step 2. Create an object
ActionScript is used to affect objects in Flash. To create a game you must create objects for the players to interact with. Depending on the guide you read, objects may be referred to as sprites, actors, or movies. For this simple game, you will create a rectangle.
- Open Flash Professional. Create a new ActionScript 3 project.
- Click the Rectangle drawing tool from the Tools panel. This panel may be in a different location, depending on the Flash Professional configuration. Draw a rectangle in the scene window.
- Select the rectangle using the Selection tool.
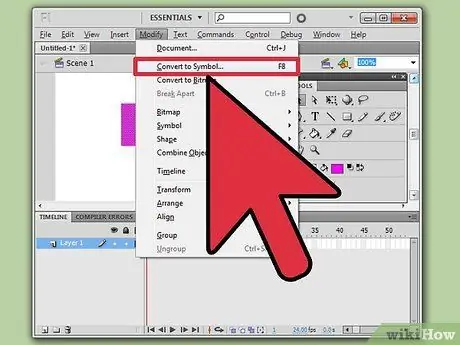
Step 3. Set the properties on the object
Select the newly selected rectangle, go to the Modify menu and select "Convert to Symbol". You can also press F8 as a shortcut. In the "Convert to Symbol" window, give the object an easily recognizable name, for example "enemy".
- Locate the Properties window. At the top of the window, there will be a blank text field labeled "Instance name" when hovering the mouse over it. Give it the same name as when you turned it into a symbol ("enemy"). This creates a unique name for interacting via the AS3 code.
- Each "event" is a separate object that the code can affect. You can copy events that have been created multiple times by clicking the Library tab and dragging them into the scene. Each time an event is added, its name changes to indicate that the object is separate ("enemy", "enemy1", "enemy2", etc.).
- When you refer to an object in code, just use the name of the event, in this case "enemy".
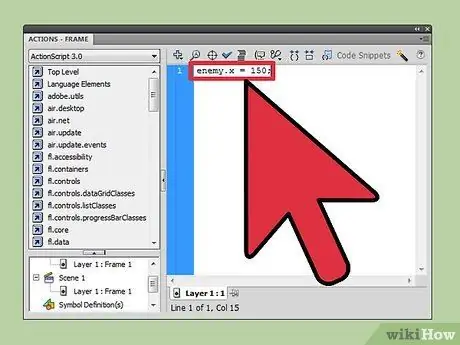
Step 4. Learn how to change the properties of an event
Once the event is created, you can set its properties via AS3. This allows you to move objects around, resize them, and so on. You can customize a property by typing the occurrence, followed by a period ".", then followed by the property, followed by the value:
- enemy.x = 150; This affects the position of enemy objects on the X axis.
- enemy.y = 150; This affects the position of enemy objects on the Y axis. The Y axis is calculated from the top of the scene.
- enemy.rotation = 45; Rotates enemy objects 45° clockwise.
- enemy.scaleX = 3; Stretches the width of the enemy object by a multiple of 3. The (-) sign will flip the object.
- enemy.scaleY = 0.5; Changes the object's height to half of its current height.
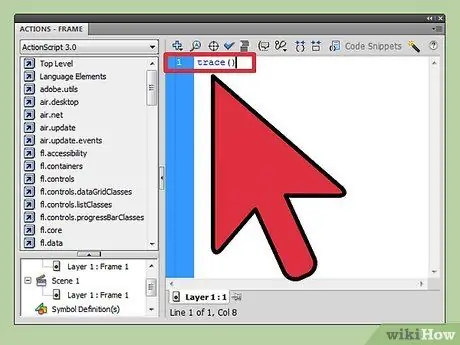
Step 5. Observe the trace() command
This command will return the current value of the specified object, and is useful for determining if everything is working as it should. You probably won't include the Trace command in your final code, but it can be useful for locating the source of the failed code.
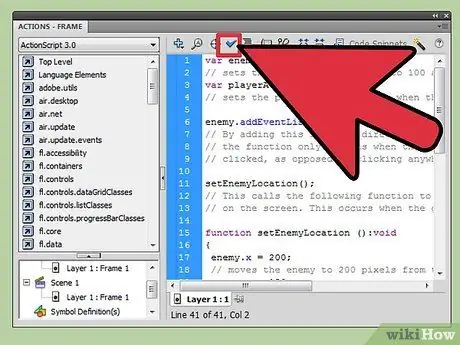
Step 6. Build the base game using the information above
You now have a basic understanding of the core functions. You can make a game where every time an enemy is clicked it will shrink in size, until the enemy is destroyed
var enemyHP:Number = 100; // Sets the enemy's HP (health) to 100 at the start of the game. var playerAttack:Number=10; // Sets the amount of the player's attack power when clicking. enemy.addEventListener(MouseEvent. CLICK, attackEnemy); // By adding this function directly to the enemy object, // this function only occurs when the object itself is // clicked, and not when it clicks anywhere on the screen. setEnemyLocation(); // Calls the following function to place the enemy // on the screen. This happens when the game starts. function setEnemyLocation():void { enemy.x = 200; // Move the enemy 200 pixels from the left of the screen enemy.y = 150; // Move the enemy 150 pixels down from the top of the screen enemy.rotation = 45; // Rotate enemy 45 degrees clockwise trace("enemy's x value is", enemy.x, "and enemy's y value is", enemy.y); // Displays the enemy's current position to find the source of the error } function attackEnemy (e:MouseEvent):void // Creates an attack function when the enemy is clicked { enemyHP = enemyHP - playerAttack; // Subtracts attack value from HP value, // Generates new HP value. enemy.scaleX = enemyHP / 100; // Change the width based on the new HP value. // The value is divided by 100 and then converted to a decimal. enemy.scaleY=enemyHP / 100; // Change height based on new HP value trace("Enemies have", enemyHP, "HP remaining"); // Display how much HP the enemy has left }
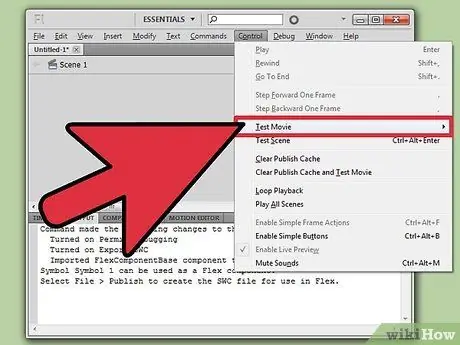
Step 7. Try it
Once you have coded, you can test this new game. Click the Control menu and select Test Movie. The game starts, and you can click on an enemy object to resize it. The Trace output will be displayed in the Output window.
Part 3 of 3: Learning Advanced Techniques
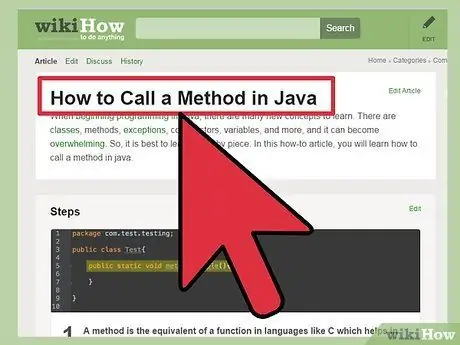
Step 1. Learn how packages work
ActionScript is Java based and uses a very similar package system. With packages you can store variables, constants, functions, and other information in separate files, and then import these files into the program. This is especially useful if you want to use packages that other people have developed that make games easier to build.
Look for a guide on Wikihow for more details on how packages work in Java
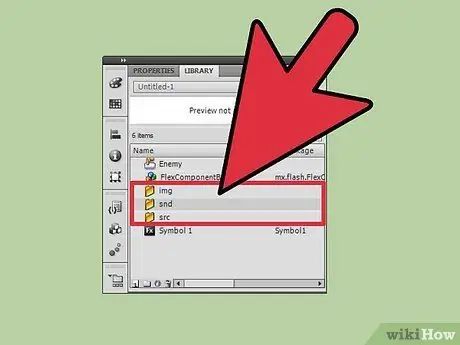
Step 2. Build the project folder
If you are creating a game that contains images and sound clips, create a folder structure in the game. You'll find it easier to store different types of elements, as well as save different packages to call.
- Create a base folder for your project. In the base folder, create an "img" folder for all art assets, a "snd" folder for all sound assets, and a "src" folder for all game and code packages.
- Create a "Games" folder in the "src" folder to store the Constants file.
- This particular structure is not required, but will make it easier to organize the work of all materials, especially for larger projects. For the simple game described above, you don't need to create any directories.
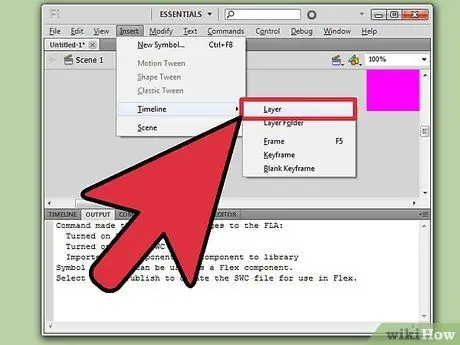
Step 3. Add sound to the game
Games without sound or music will quickly bore players. You can add sound to objects using the Layers tool.
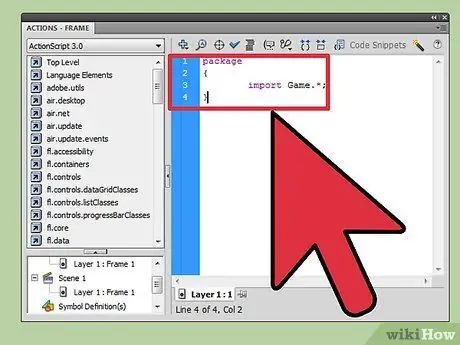
Step 4. Create the Constants file
If your game has a lot of values that will stay the same throughout the game, you can create a Constants file to collect them all in one place so they can be easily summoned. Constants can include values such as gravity, player speed, and other values that may need to be called repeatedly.
-
If you created a Constants file, place it in a folder in the project and then import it as a package. Assume that you create a Constants.as file and place it in the game folder. To import it, use the following code:
package { import Game.*; }
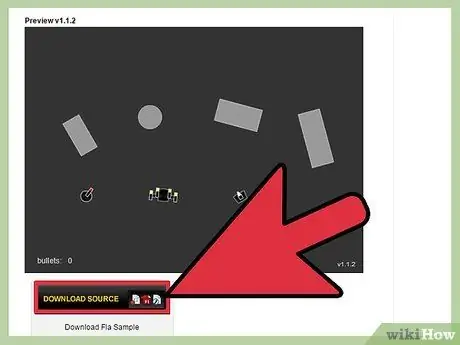
Step 5. Look at other people's play
While many developers won't disclose their game code, there are a variety of tutorial projects and other open source projects that will allow you to view code and interact with game objects. This is a great way to learn a variety of advanced techniques that can help your game stand out.