Java is an object-oriented programming language created in 1995 by James Gosling. That is, the language presents concepts as "objects" with "fields" (i.e. attributes that describe objects) and "methods" (actions that objects can perform). Java is a "once written, run anywhere" language. That is, the language is designed to run on any platform that has a Java Virtual Machine (JVM). Because Java is a very long-winded programming language, it is easy for beginners to learn and understand. The following tutorial is an introduction to writing programs with Java.
Step
Method 1 of 3: Writing Prime Java Programs
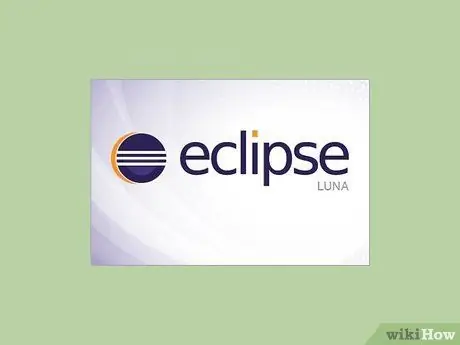
Step 1. To start writing programs with Java, define your work environment
Many programmers use Integrated Development Environment (IDE) such as Eclipse and Netbeans for Java programming, but we can write Java programs and compile them without an IDE.
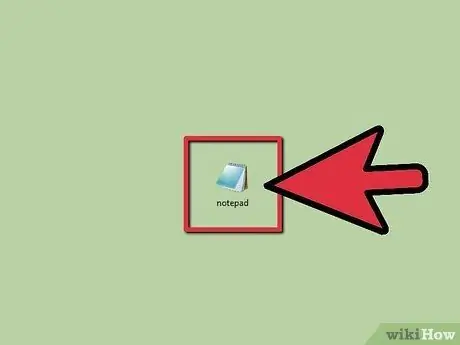
Step 2. Any type of program similar to Notepad will suffice for programming with Java
Hardliners sometimes prefer text editors built into the terminal, such as vim and emacs. A powerful text editor that can be installed on both Windows and Linux-based computers (Mac, Ubuntu, etc.) is Sublime Text. It is this text editor that we will be using in this tutorial.
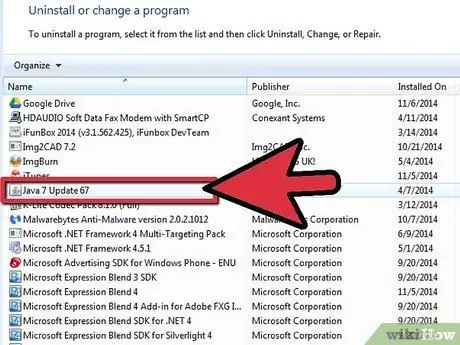
Step 3. Make sure you have the Java Software Development Kit installed
You will need it to compile your program.
-
On Windows-based systems, if the environment variables do not match, you may experience failure while running
javac
- . Read the article How to Install the Java Software Development Kit for more details on installing the JDK to avoid this error.
Method 2 of 3: Hello World Program
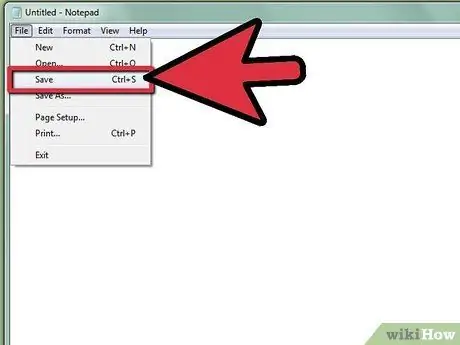
Step 1. First of all, we will create a program that displays the message "Hello World
"In your text editor, create a new file and save it with the name "HelloDunia.java". HelloDunia is your class name and the class name must be the same as your file name.
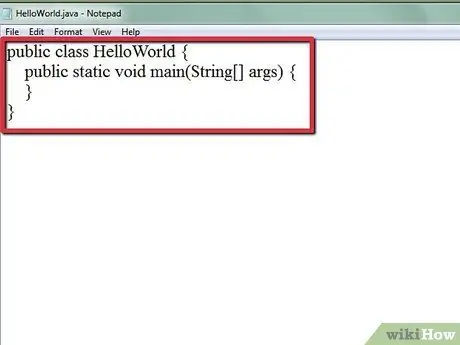
Step 2. Define the main class and methods
Main method
public static void main(String args)
is a method that will be executed while the program is running. This main method will have the same method declaration in all Java programs.
public class HelloWorld { public static void main(String args) { } }
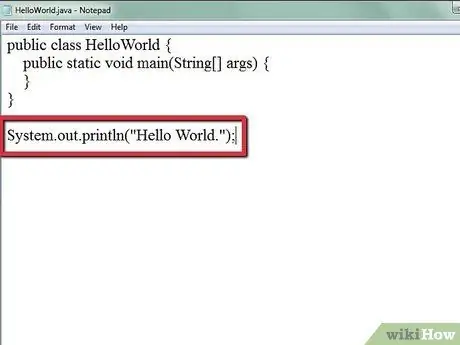
Step 3. Write a line of code that will display "Hello World
System.out.println("Hello World.");
-
Pay attention to the components of this line:
-
System
- commands the system to do something.
-
out
- tells the system that we will produce output.
-
println
- short for "print line". So, we instruct the system to display the line in the output.
-
Quotation marks on
("Hello World.")
means method
System.out.println()
pass in a parameter, which, in this case, is a String
"Hello World."
-
-
Note that there are several rules in Java that must be adhered to:
- You should always add a semicolon (;) at the end of each line.
- Java is case sensitive. So you must write the method name, variable name, and class name in correct letters or you will fail.
- Blocks of code that are specific to a particular method or loop are enclosed in curly braces.
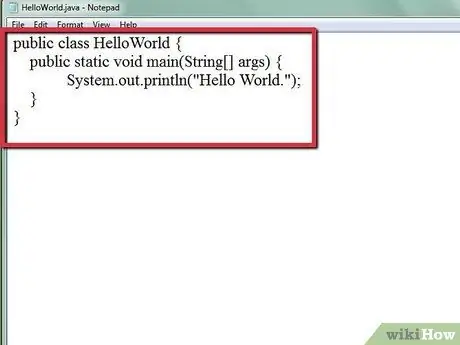
Step 4. Put everything together
Your final Halo World program should look like the following:
public class HelloWorld { public static void main(String args) { System.out.println("Hello World."); } }
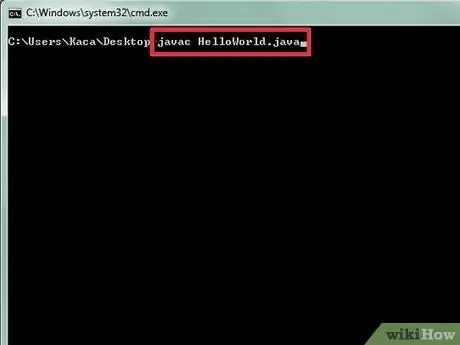
Step 5. Save your file and open a command prompt or terminal to compile the program
Go to the folder where HaloDunia.java is saved and type in
javac HelloDunia.java
. This command tells the Java compiler that you want to compile HaloDunia.java. If an error occurs, the compiler will tell you what went wrong. Otherwise, you won't see any messages from the compiler. If you look at the directory where you currently have HaloDunia.java stored, you will see HaloDunia.class. This is the file that Java will use to run your program.
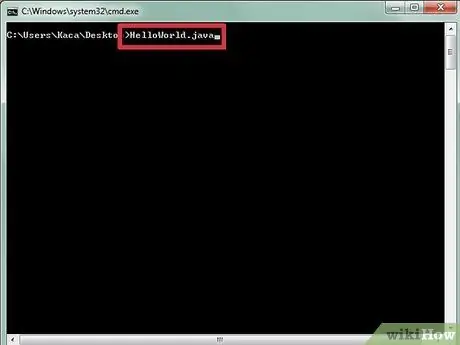
Step 6. Run the program
Finally, we will run our program! In the command prompt or terminal, type
java HelloWorld
. This command tells Java that you want to run the HaloWorld class. You will see "Hello World." appears on the console.
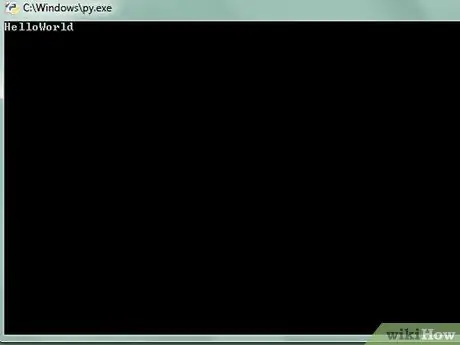
Step 7. Congratulations, your first Java program is ready
Method 3 of 3: Input and Output
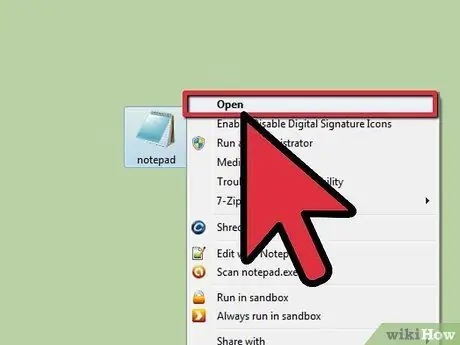
Step 1. We will now expand the Hello World program to include user input
In the Hello World program, we display a string for the user to see, but the interactive part of the program is when the user has to enter input into the program. We will now extend the program to ask the user to enter their name and then greet the user by that name.
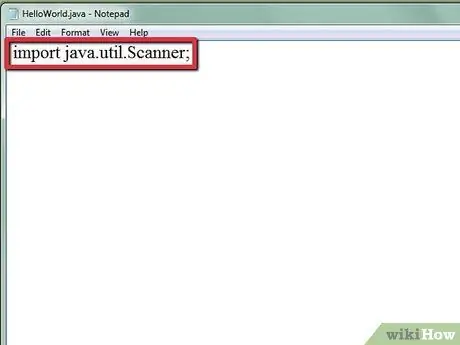
Step 2. Import the Scanner class
In Java, we have some kind of built-in library that we can access, but we have to import it first. One of these libraries is java.util, which contains the Scanner object that we need to get input from the user. To import the Scanner class, add the following line at the beginning of the code.
import java.util. Scanner;
- This code tells the program that we want to use the Scanner object that is in the java.util package.
-
If we want to access every object in the java.util package, just write
import java.util.*;
- at the beginning of the code.
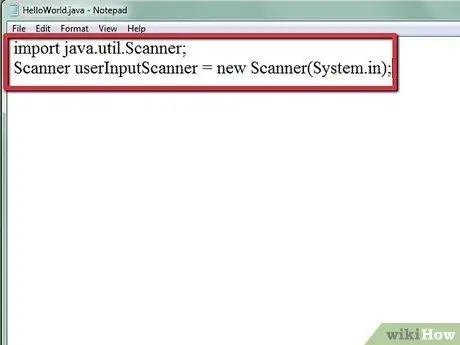
Step 3. Inside the main method, instantiate a new instance of the Scanner object
Java is an object-oriented programming language. So, this language describes the concept of using objects. The Scanner object is an example of an object that has fields and methods. To use the Scanner class, we must create a new Scanner object whose fields we can fill in and methods we can use. To do this, write:
Scanner userInputScanner = new Scanner(System.in);
-
userInputScanner
- is the name of the Scanner object we just sampled. Note that the name is written in upper and lower case; this is a variable naming convention in Java.
-
We use operator
new
to create a new object instance. So, in this example, we create a new instance of the Scanner object by writing
new Scanner(System.in)
- .
-
The Scanner object includes parameters that tell the object what to scan. In this case, we enter
System.in
as parameters.
System.in
- tells the program to scan for input from the system, i.e. the input the user will type into the program.
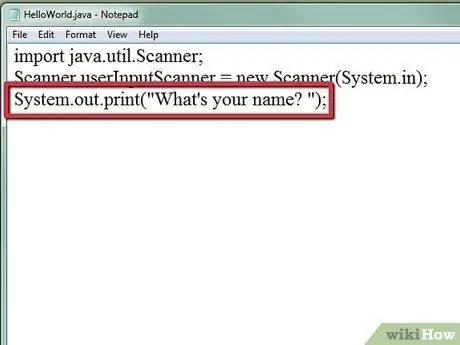
Step 4. Ask for input from the user
We have to request input from the user so that the user knows when to type something into the console. This step can be taken by
System.out.print
or
System.out.println
System.out.print("What is your name?");
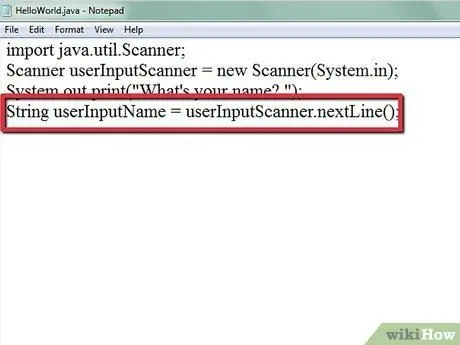
Step 5. Ask the Scanner object to enter the next line the user typed and store it in a variable
Scanner will always enter data containing what the user typed. The following line will ask Scanner to take the name the user typed in and store it in a variable:
String userInputName = userInputScanner.nextLine();
-
In Java, the convention for using methods of an object is
objectName.methodName(parameters)
. In
userInputScanner.nextLine()
we call the Scanner object with the name we just gave it then we call its method
nextLine()
- which does not include any parameters.
-
Notice that we store the next line in another object: a String object. We have named our String object
userInputName
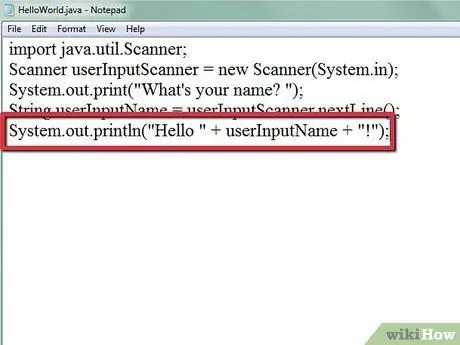
Step 6. Show the greeting to the user
Now that we have saved the username, we can display the greeting to the user. Remember with
System.out.println("Hello World.");
that we write in the main class? All the code we just wrote should be above that line. Now we can modify that line to be:
System.out.println("Hello " + userInputName + "!");
-
The way we concatenate "Hello ", username, and "!" by writing
"Hello " + userInputName + "!"
- called String concatenation.
- Here we have three strings: "Hello ", userInputName, and "!". Strings in Java are fixed, meaning they cannot be changed. So, when we concatenate these three strings, we are basically creating a new string containing the greeting.
-
Then we take this new string and pass it as a parameter into
System.out.println
- .
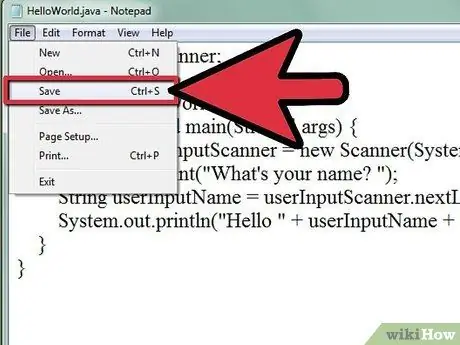
Step 7. Arrange everything and save
Our code will now look like this:
import java.util. Scanner; public class HelloWorld { public static void main(String args) { Scanner userInputScanner = new Scanner(System.in); System.out.print("What is your name?"); String userInputName = userInputScanner.nextLine(); System.out.println("Hello " + userInputName + "!"); } }
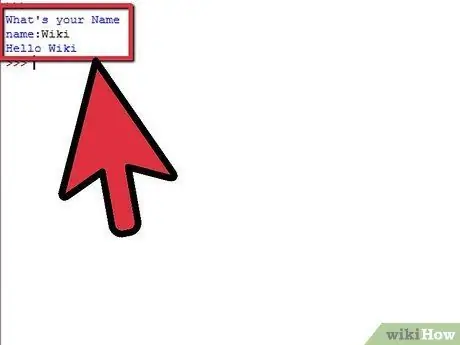
Step 8. Compile and run
Go to the command prompt or terminal and run the same command as we run HaloDunia.java. We must first compile the program:
javac HelloDunia.java
. Then we can run it:
java HelloWorld
Tips
- Java is an object-oriented programming language. So it's a good idea to read up on the basics of object-oriented programming languages to find out more.
-
Object-oriented programming has many special features. Three of them are:
- Encapsulation: ability to restrict access to some component objects. Java has private, protected, and public modifiers for fields and methods.
- Polymorphism: the object's ability to use multiple identities. In Java, an object can be inserted into another object to use the methods of that other object.
- Legacy: the ability to use fields and methods from other classes in the same hierarchy as the current object.