There are many ways to compare two dates in the Java programming language. In computers, a date is represented by a number (the data type Long) in units of time -- that is, the number of milliseconds that have elapsed since January 1, 1970. In Java, Date is an object, which means it has several methods for making comparisons. Any method used to compare two dates is essentially comparing the time units of the two dates.
Step
Method 1 of 4: Using compareTo
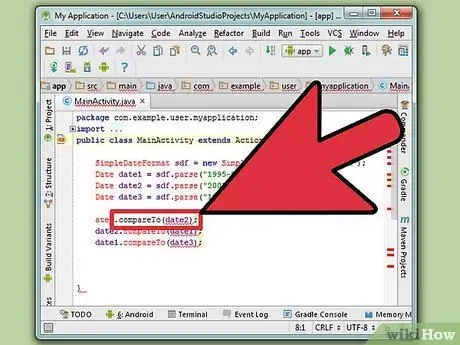
Step 1. Use compareTo
The Date object implements Comparable so 2 dates can be compared with each other directly with the compareTo method. If both dates have the same number in time units, then the method returns zero. If the second date is less than the first, a value less than zero is returned. If the second date is greater than the first, the method returns a value greater than zero. If both dates are the same, then the method will return a null value.
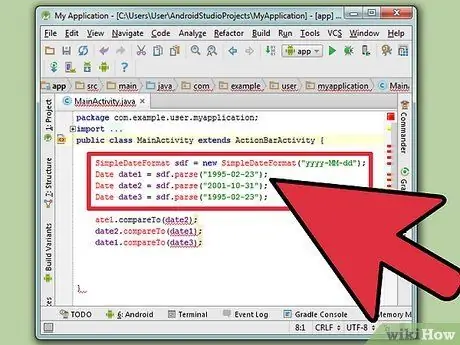
Step 2. Create multiple Date objects
You must create multiple Date objects before comparing them. One of the easiest ways to do this is to use the SimpleDateFormat class. This class makes it easy to convert an input date value into a Date object.
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"). To declare a value in a new ''Object Date'', use the same date format when creating the date. Date date1 = sdf.parse("1995-02-23"); //date1 is February 23, 1995 Date date2 = sdf.parse("2001-10-31"); //date2 is October 31, 2001 Date date3 = sdf.parse("1995-02-23"); //date3 is February 23, 1995
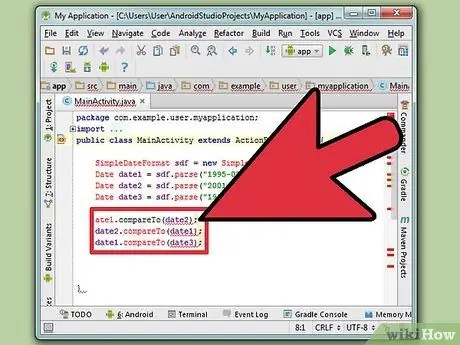
Step 3. Compare the Date objects
The following code will show you examples for each case -- less than, equal, and greater than.
date1.compareTo(date2); //date1 < date2, returns a value less than 0 date2.compareTo(date1); //date2 > date1, returns a value greater than 0 date1.compareTo(date3); //date1 = date3, so it will output 0 on console
Method 2 of 4: Using Equals, After and Before
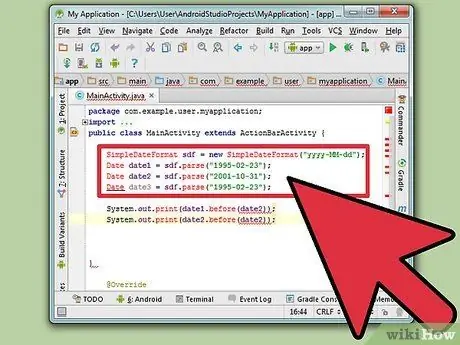
Step 1. Use equals, after and before
Dates can be compared using the equals, after, and before methods. If two dates have the same value in time, the equals method returns true. The following example will use the Date object created in the example compareTo method.
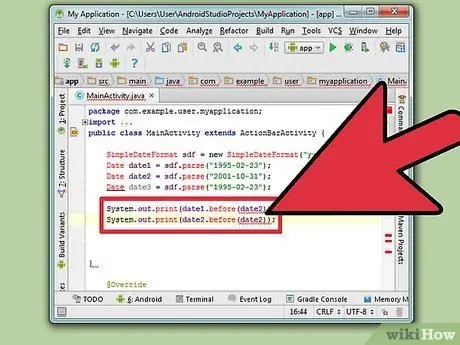
Step 2. Compare with the before method
The following code shows an example case that returns true and false. If date1 is a date before date2, the before method returns true. Otherwise, the before method returns false.
System.out.print(date1.before(date2)); // display the value ''true'' System.out.print(date2.before(date2)); // return the value ''false''
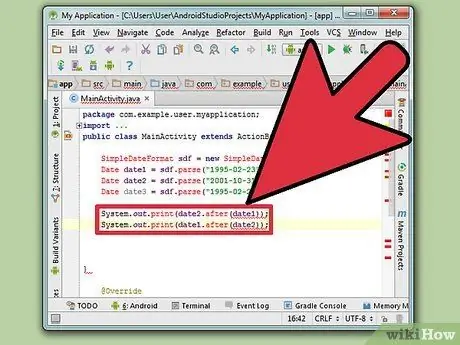
Step 3. Compare using the after method
The following code shows an example case that returns true and false. If date2 is the date after date1, the after method returns true. Otherwise, the after method will return false.
System.out.print(date2.after(date1)); // display the value ''true'' System.out.print(date1.after(date2)); // display the value ''false''
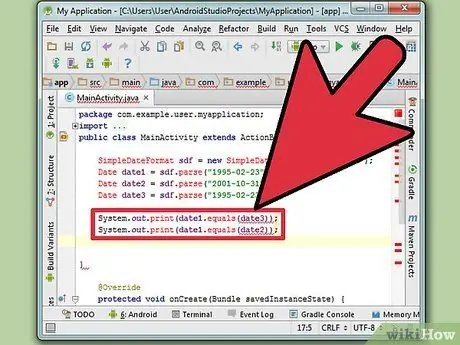
Step 4. Compare with equals method
The following code shows an example case that returns true and false. If both dates are equal, the equals method returns true. Otherwise, the equals method returns false.
System.out.print(date1.equals(date3)); // display the value ''true'' System.out.print(date1.equals(date2)); // display the value ''false''
Method 3 of 4: Using Class Calendar
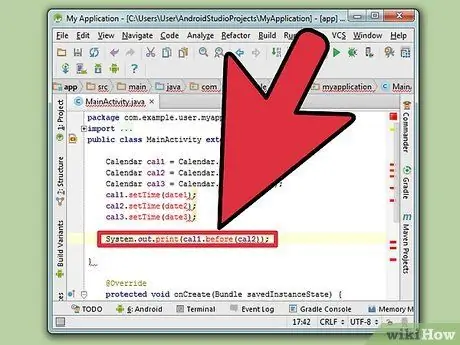
Step 1. Use Class Calendar
The Calendar class also has compareTo, equals, after, and before methods that work the same as those described earlier for the Date class. So if the date information is stored in a Class Calendar, you don't need to extract the date just to do a comparison.
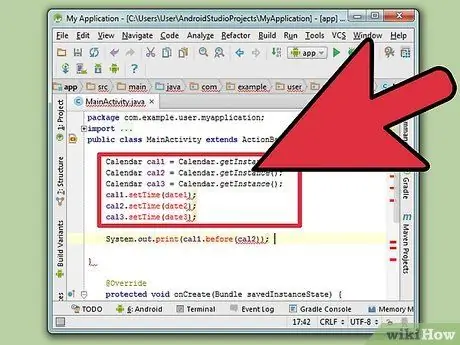
Step 2. Create an instance of Calendar
To use methods in Class Calendar, you must create multiple Calendar instances. Fortunately, you can use the value of a previously created Date instance.
Calendar cal1 = Calendar.getInstance(); //declare cal1 Calendar cal2 = Calendar.getInstance(); //declare cal2 Calendar cal3 = Calendar.getInstance(); //declare cal3 cal1.setTime(date1); //put the date into cal1 cal2.setTime(date2); cal3.setTime(date3);
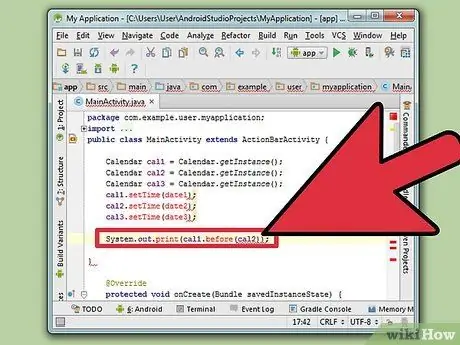
Step 3. Compare cal1 and cal2 using the before method
The following code will output the value of tr
System.out.print(cal1.before(cal2)); //will return value ''true''
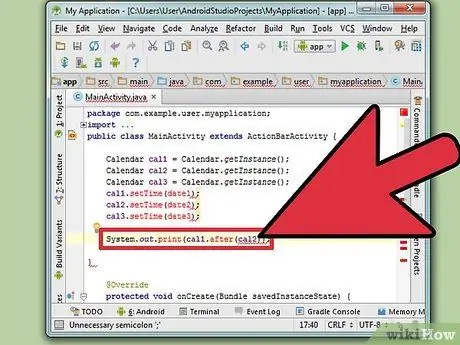
Step 4. Compare cal1 and cal2 using the after method
The following code will return false because cal1 is the date before cal2.
System.out.print(cal1.after(cal2)); // return the value ''false''
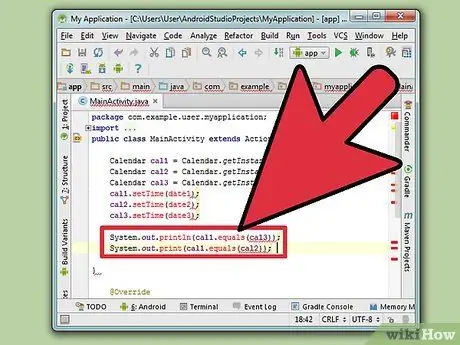
Step 5. Compare cal1 and cal2 using the equals method
The following code will show an example case that returns true and false. The state depends on the Calendar instance being compared. The following code will return the value "true", then "false" on the next line.
System.out.println(cal1.equals(cal3)); // return the value ''true'': cal1 == cal3 System.out.print(cal1.equals(cal2)); // return the value ''false'': cal1 != cal2
Method 4 of 4: Using getTime
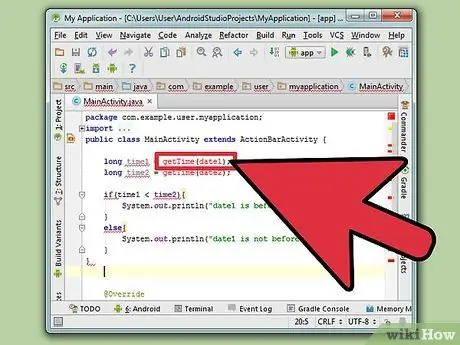
Step 1. Use getTime
You can also directly compare the time unit values of two dates, although the previous two methods may be easier to read and prefer. This way you'll be comparing 2 primitive data types, so you can use the operands "", and "==".
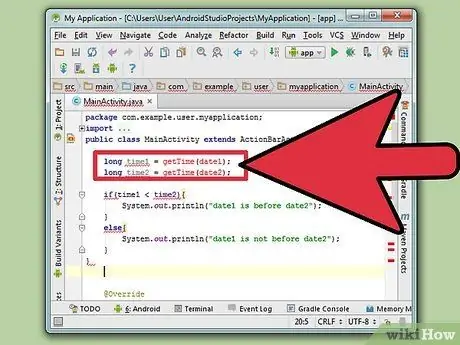
Step 2. Create a time object in Long number format
Before you can compare dates, you must create a Long Integer value from the previously created Date object. Luckily, the getTime() method will do it for you.
long time1 = getTime(date1); //declare primitive time1 of date1 long time2 = getTime(date2); //declare primitive time2 value of date2
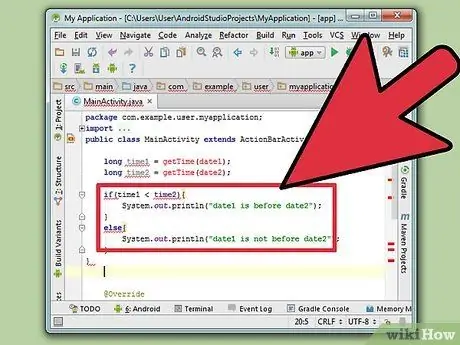
Step 3. Perform a less than comparison
Use the less than (<) operand to compare these two integer values. Since time1 is less than time2, the first message will appear. The else statement is included to complete the syntax.
if(time1 < time2){ System.out.println("date1 is the date before date2"); //will show because time1 < time2 } else{ System.out.println("date1 is not a date before date2"); }
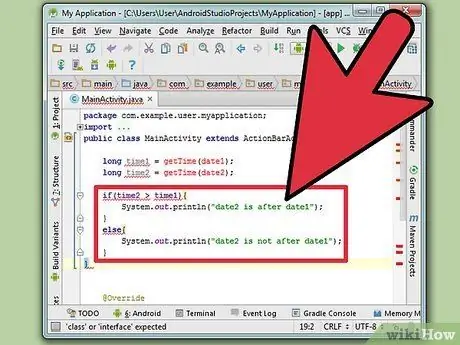
Step 4. Perform a greater than comparison
Use the greater than (>) operand to compare these two integer values. Because time1 is greater than time2, the first message will appear. The else statement is included to complete the syntax.
if(time2 > time1){ System.out.println("date2 is the date after date1"); //will show because time2 > time1 } else{ System.out.println("date2 is not the date after date1"); }
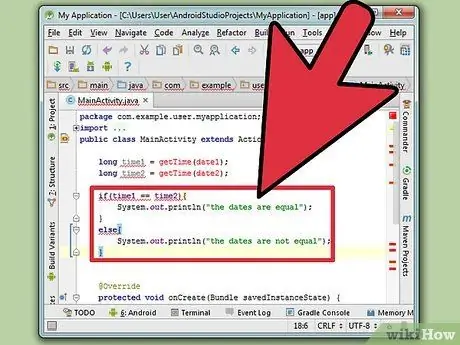
Step 5. Perform an equals comparison
Use the operand function to check the equality of values (==) to compare these two integers. Since time1 is equal to time3, the first message will appear. If the program flow goes into the else statement, then it means that the two times don't have the same value.
if(time1 == time2){ System.out.println("both dates are the same"); } else{ System.out.println("The 1st is not the same as the 2nd"); //will show because time1 != time2 }