Comparing string lengths is a commonly used function in C programming, because it can tell you which string has more characters. This function is very useful in sorting data. Comparing strings requires a special function; don't use != or ==.
Step
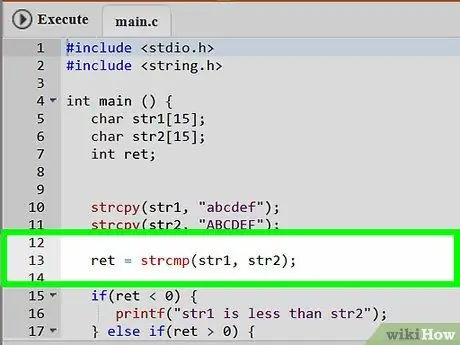
Step 1. There are 2 types of functions that you can use to compare strings in C language
Both of these functions are included in the library.
- strcmp(): This function compares two strings and returns the result of comparing the number of characters between them.
- strncmp(): This function is the same as strcmp(), except it compares the first n} characters in the string. This function is considered safer because it prevents the program from stalling due to overload.
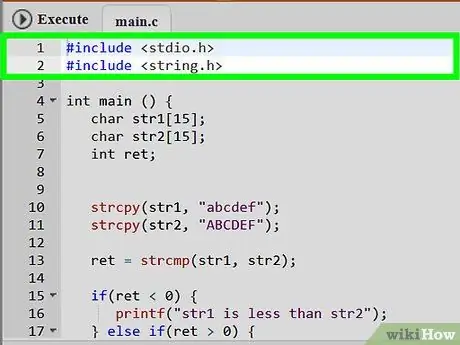
Step 2. Run the program with the libraries you need
We recommend that you run and, along with any other libraries you need for a particular program.
#include #include
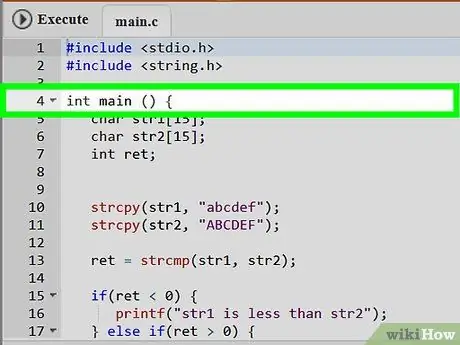
Step 3. Run a function
int. This is the easiest way to learn this function, because it returns the integer value of the comparison of the number of characters in two strings.
#include #include int main() { }
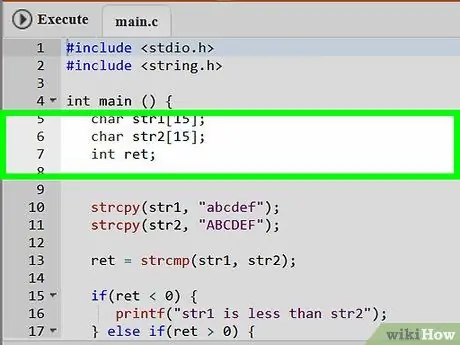
Step 4. Define the two strings you want to compare
For example, we will compare 2 strings of type char data that have been defined previously. You can also define the value returned by this function to have the data type integer.
#include #include int main() { char *str1 = "apple"; char *str2 = "orange"; int ret; }
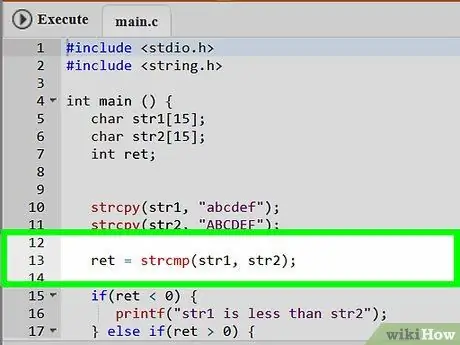
Step 5. Add comparison function
Once you've defined those two strings, you can add a comparison function. We'll be using strncmp(), so we'll need to make sure that the number of characters to measure is set up in the function.
#include #include int main() { char *str1 = "apple"; char *str2 = "orange"; int ret; ret = strncmp(str1, str2, 6); /*This function will compare both ''string'' for 6 characters */ }
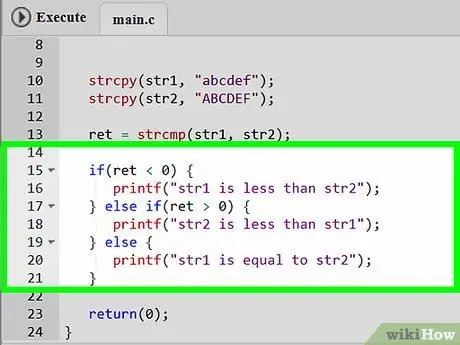
Step 6. Use statements
If…Else to do the comparison. After you add a function to your program, you can use a statement to display which string has more characters. strncmp() will return 0 if the strings have the same number of characters, a positive number if str1 is longer and a negative number if str2 is longer.
#include #include int main() { char *str1 = "apple"; char *str2 = "orange"; int ret; ret = strncmp(str1, str2, 6); if(ret > 0) { printf("str1 is longer"); } else if(ret < 0) { printf("str2 is longer"); } else { printf("Both strings are the same length"); } return(0); }