C is a fairly old programming language. C was developed in the 70s, but it's still pretty powerful because C runs at a low level. Learning C is a great way to introduce you to more complex programming languages, and the knowledge you have can be applied to almost any programming language and help you understand application development. To start learning the C programming language, see step 1 below.
Step
Part 1 of 6: Preparation
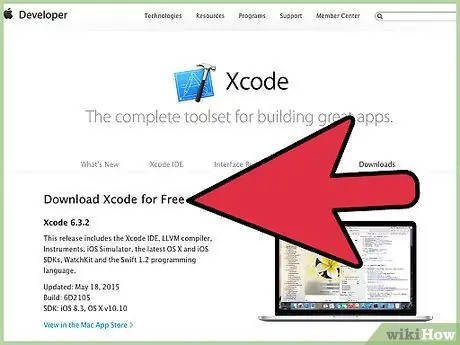
Step 1. Download and install the C compiler
C code must be compiled with a program that interprets the code into signals that the machine understands. Compilers are usually free, and various compilers are available for different operating systems.
- For Windows, try Microsoft Visual Studio Express or mingw.
- For Mac, XCode is one of the best C compilers.
- For Linux, gcc is one of the most popular options.
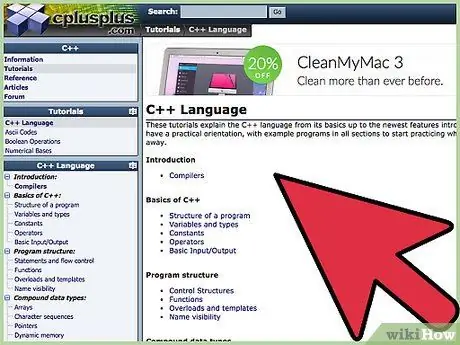
Step 2. Understand programming basics
C is a fairly old programming language and can be very powerful. C was designed for Unix operating systems, but has been developed for almost all operating systems. The modern version of C is C++.
Basically, C is composed of functions, and in those functions, you can use variables, conditional statements, and loops to store and manipulate data
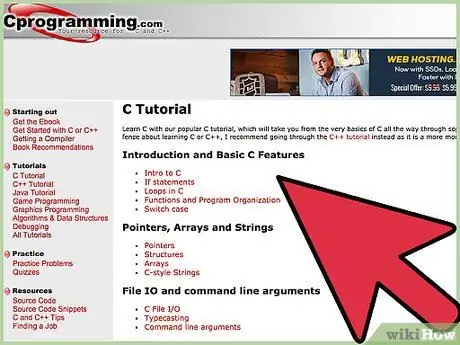
Step 3. Read the basic code
Take a look at the following basic programs to find out how various aspects of programming languages work, and to get an idea of how programs work.
include
int main() { printf("Hello, World!\n"); getchar(); returns 0; }
- The #include function is used before the program starts, and loads the libraries that have the functionality you need. In this program, stdio.h lets you use the printf() and getchar() functions.
- The int main() function tells the compiler that the program is executing a function named "main" and will return an integer when it's done. All C programs perform the "main" function.
- {} indicates that all the code in it is part of the function. In this program, all the code in it is included in the "main" function.
- The printf() function returns the content in quotes to the user's screen. Quotation marks are used so that the text is printed correctly. \n tells the compiler to move the cursor to a new line.
- ; marks the end of the line. Almost all lines of C code must end with a semicolon.
- The getchar() command tells the compiler to wait for keyboard input before continuing. This is useful because many compilers will run the program and immediately close the window. This function prevents the program from finishing before a key is pressed.
- The return 0 command signifies the end of the function. Note that the "main" function is an int function. That is, "main" needs to return an integer after the program finishes. Zero indicates the program was executed correctly; another number indicates the program has encountered an error.
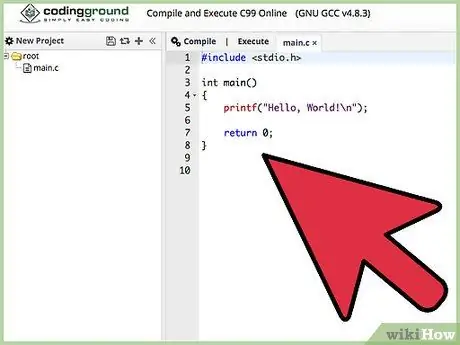
Step 4. Try compiling the program
Enter the program in your code editor and save it as a "*.c" file. Compile by pressing the Build or Run button.
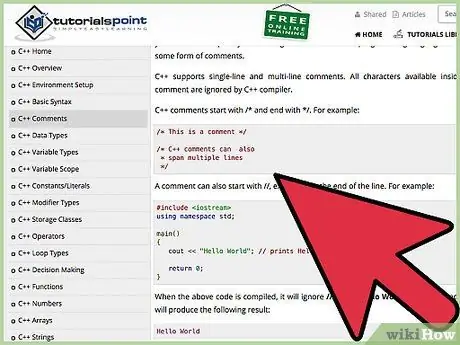
Step 5. Always comment your code
Comments are pieces of code that don't compile, but allow you to explain what's going on. Comments are useful for reminding yourself of the functionality of your code, and for helping other developers who might see your code.
- To comment code in C, put /* at the beginning of the comment and */ at the end of the comment.
- Comment out all sections of code except the most basic.
- Comments can be used to exclude certain sections of code without deleting them. Uncomment the code you want to exclude and compile the program. If you want to return the code, uncomment it.
Part 2 of 6: Using Variables
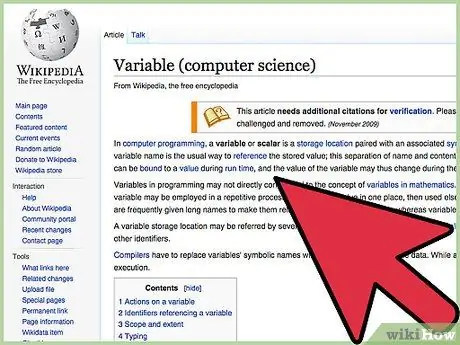
Step 1. Understand the function of variables
Variables allow you to store data, either from calculations in the program or user input. Variables must be defined before they can be used, and there are several types of variables to choose from.
Variables that are quite widely used are int, char, and float. Each type of variable stores a different type of data
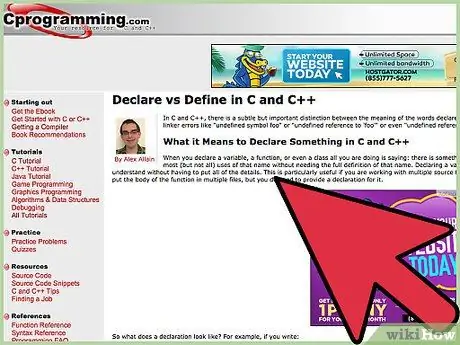
Step 2. Learn how to declare variables
Variables must be created, or declared, before they can be used by the program. Declare a variable by entering the data type and variable name. For example, the following variables can be used:
floats x; charnames; int a, b, c, d;
- Remember that you can declare multiple variables in a row, as long as they are of the same type. Separate the name of each variable with a comma.
- Like most lines in C, each variable needs to end with a semicolon.
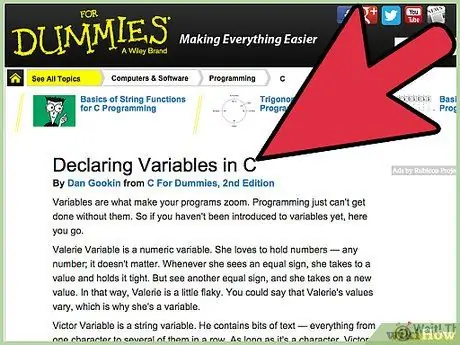
Step 3. Know where you can declare variables
Variables must be declared at the beginning of each code block (inside {}). If you try to declare variables later, your program will not run correctly.
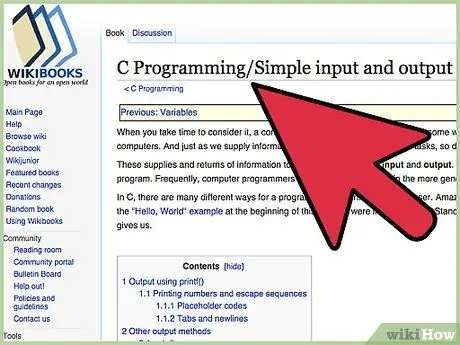
Step 4. Use variables to store user input
Once you understand how variables work, you can write programs that store user input. You will use the scanf function in your program. This function searches for a given input at a specified value.
include
int main() { int x; printf("Enter a number: "); scanf("%d", &x); printf("You entered %d", x); getchar(); returns 0; }
- The line "%d" tells scanf to look for an integer in user input.
- The & before the x variable tells scanf where the variable should be found to change it, and stores an integer in the variable.
- The last printf command returns an integer to the user.
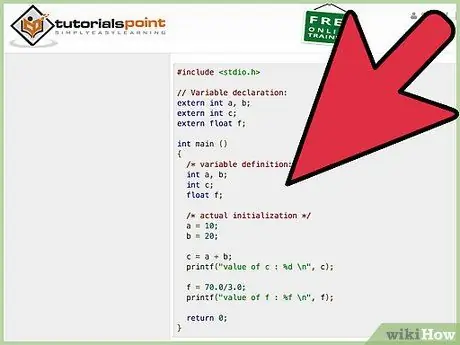
Step 5. Manipulate your variables
You can use mathematical expressions to modify data that is already stored in a variable. The difference in mathematical expressions you should understand is that = sets the value of a variable, while == compares the values of both sides to see if they are similar.
x = 3 * 4; /* set "x" to 3 * 4, or 12 */ x = x + 3; /* adds 3 to the original "x" value, and sets the new value as a variable */ x == 15; /* checks if "x" is equal to 15 */ x < 10; /* check if the value of "x" is less than 10 */
Part 3 of 6: Using Conditional Statements
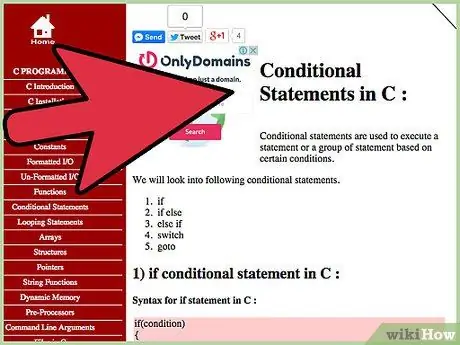
Step 1. Understand the basics of conditional statements
Conditional statements are at the heart of many programs, and are statements whose answers are TRUE or FALSE, then execute the program based on the result. The most basic conditional statement is if.
TRUE and FALSE work in different ways in C. TRUE always ends in a number other than 0. When you perform a comparison, if the result is TRUE, the number "1" will be output. If "FALSE", "0" will exit. Understanding this will help you understand how IF statements are processed
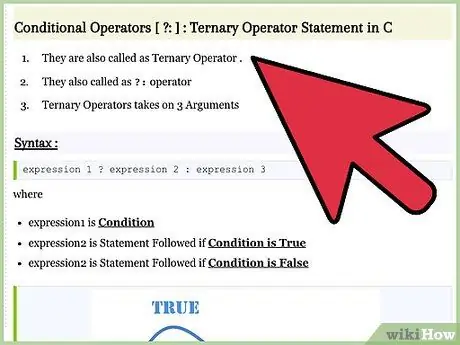
Step 2. Learn the basic conditional operators
Conditional commands use mathematical operators to compare values. This list contains the most frequently used conditional operators.
/* greater than */ < /* less than */ >= /* greater than or equal to */ <= /* less than or equal to */ == /* equal to */ != /* not equal to */
10 > 5 TRUE 6 < 15 TRUE 8 >= 8 TRUE 4 <= 8 TRUE 3 == 3 TRUE 4 != 5 TRUE
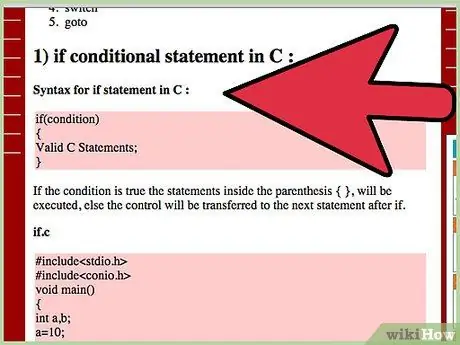
Step 3. Write a basic IF statement
You can use an IF statement to specify what the program will do after a statement is checked. You can combine it with other conditional commands to make a great multiple-option program, but this time, create a basic IF statement to get used to.
include
int main(){ if (3 < 5) printf("3 is less than 5"); getchar();}
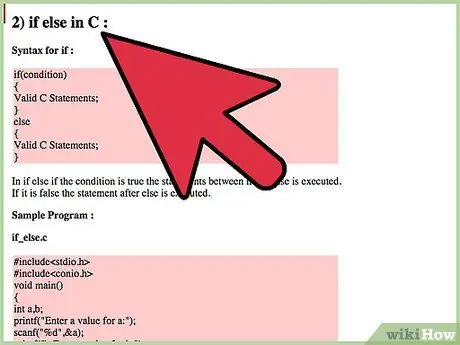
Step 4. Use ELSE/IF statements to develop your condition
You can extend the IF statement by using ELSE and ELSE IF to handle different results. The ELSE statement will be executed if the IF statement evaluates to FALSE. ELSE IF lets you include multiple IF statements in a single block of code to handle different cases. Read the following example to see how conditional statements interact.
#include int main() { int age; printf("Please enter your current age: "); scanf("%d", &age); if (age <= 12) { printf("You're just a kid!\n"); } else if (age < 20) { printf("Being a teenager is pretty great!\n"); } else if (age < 40) { printf("You're still young at heart!\n"); } else { printf("With age comes wisdom.\n"); } return 0; }
The program takes the input from the user and takes it through the IF statements. If the number satisfies the first statement, then the first printf statement is returned. If it does not satisfy the first statement, it is taken through each ELSE IF statement until it finds one that works. If it doesn't match any of them, it goes through the ELSE statement at the end
Part 4 of 6: Learning Loops
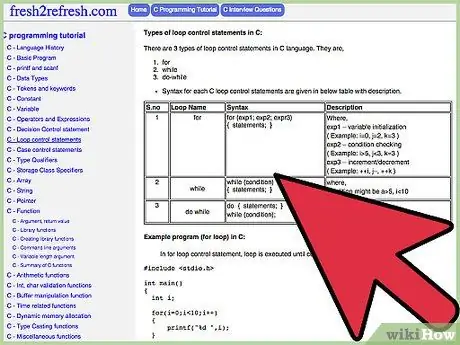
Step 1. Understand how loops work
Loops are one of the most important aspects of programming, as they allow you to repeat blocks of code until specific conditions are met. This can make repeating actions very easy to implement, and keeps you from having to write new conditional statements each time you want something to happen.
There are three main types of loops: FOR, WHILE, and DO…WHILE
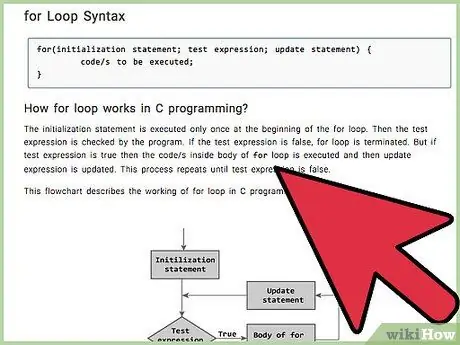
Step 2. Use a FOR loop
This is the most common and useful loop type. It will continue running the function until the conditions set in the FOR loop are met. FOR loops require three conditions: initializing the variable, the conditions to be met, and the way the variable is updated. If you don't need all of these conditions, you will still need to leave a blank space with a semicolon, otherwise the loop will run forever.
include
int main(){ int y; for (y = 0; y < 15; y++;){ printf("%d\n", y); } getchar();}
In the above program, y is 0, and the loop will continue as long as the y value is below 15. Each time the y value is displayed, the y value will be added by 1 and will continue to repeat itself. Once y reaches 15, the loop will stop
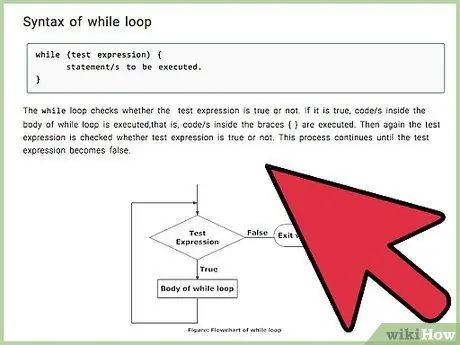
Step 3. Use the WHILE loop
The WHILE loop is simpler than the FOR loop, because it only has one condition and will iterate over as long as the condition is true. You don't need to start or update variables, although you can do that in the core loop.
#include int main() { int y; while (y <= 15){ printf("%d\n", y); y++; } getchar(); }
The y++ command adds 1 to the y variable each time the loop is executed. Once y reaches 16 (remember that this loop will run as long as y is less than or equal to 15), the loop will stop
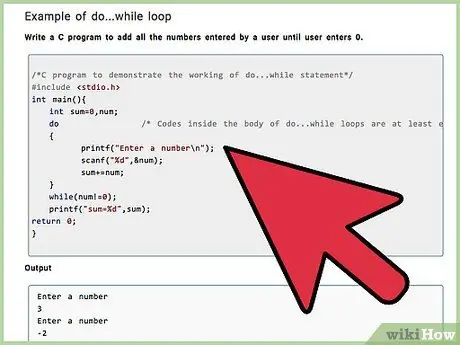
Step 4. Use the "DO
.. WHILE . This loop is useful if you want to ensure that the loop is executed at least once. In the FOR and WHILE loops, the loop condition is checked at the beginning of the loop, allowing the condition not to be met and the loop to fail. The DO…WHILE loop checks the condition at the end loop, which ensures the loop is executed at least once.
#include int main() { int y; y = 5; do { printf("This loop is running!\n"); } while (y != 5); getchar(); }
- This loop will display a message even if the condition is FALSE. The variable y is set to 5 and the loop is set to run when y is not equal to 5, so the loop stops. The message was printed because the condition was not checked until the end of the program.
- The WHILE loop in the DO…WHILE package must end with a semicolon. This case is the only case where the loop ends with a semicolon.
Part 5 of 6: Using Functions
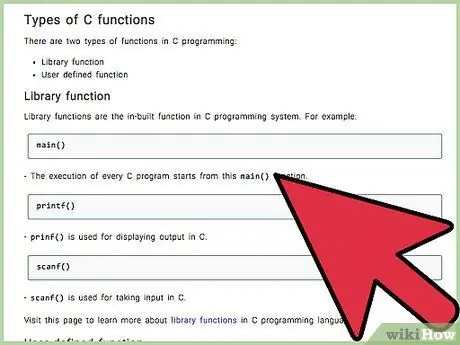
Step 1. Understand the basics of functions
Functions are pieces of code that can be called from other parts of the program. Functions allow you to repeat code easily, and make programs easier to read and modify. You can use all of the techniques in this article in a function, and even use other functions.
- The main() line at the top of this entire example is a function, as is getchar()
- The use of functions is essential for efficient and readable code. Use the best possible functions to create a neat program.
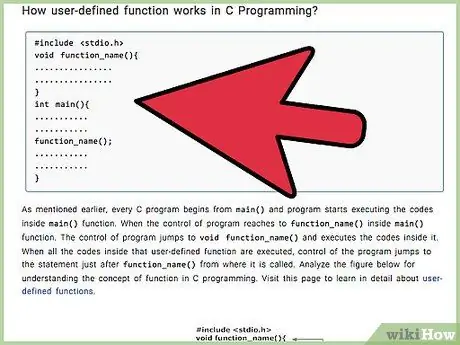
Step 2. Start with an outline
Functions should be created after you have outlined their use before starting to program. The basic syntax of a function is "return_type name (argument1, argument2, etc.);". For example, to create a function that adds two numbers:
int add(int x, int y);
This code will create a function that adds two integers (x and y) and then returns the result as an integer
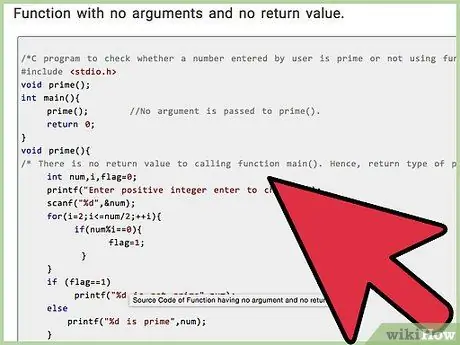
Step 3. Use a function in a program
You can use the program outline to create a program that accepts two integer inputs from the user and then adds them. The program will control how the increment function works and use it to change the entered number.
#include int add(int x, int y); int main() { int x; int y; printf("Enter two numbers to add together: "); scanf("%d", &x); scanf("%d", &y); printf("The sum of your numbers is %d\n", add(x, y)); getchar(); } int add(int x, int y) { return x + y; }
- Note that the program outline is located at the top. This outline tells the compiler what to do when the function is called and the result of the function. This outline is only useful if you want to define functions in other parts of the program. You can define add() before main(), and the result will be the same.
- The actual function of a function is defined at the bottom of the program. The main() function accepts integer input from the user and passes it to the add() function for processing. The add() function returns the result to main()
- Once add() is defined, the function can be called anywhere in the program.
Part 6 of 6: Continuing the Lesson
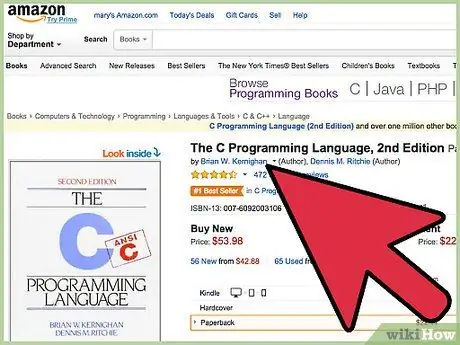
Step 1. Find some C textbooks
This article covers the basics of C programming, but only covers the surface. A good reference book will help you solve problems and help you overcome confusion.
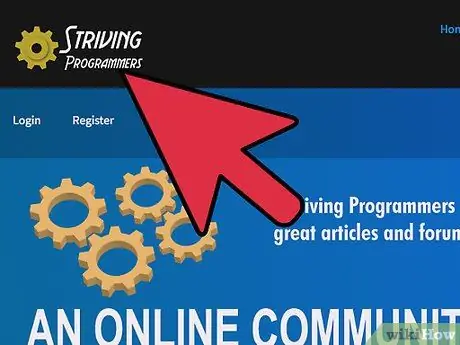
Step 2. Join the community
Many communities, both online and offline, are dedicated to programming and programming languages. Find other C programmers to exchange ideas and code with, and you'll learn a lot too.
Attend hackathon events whenever possible. It is an event where teams and programmers race against time to program and solve problems, often producing creative results. You can find many talented programmers at this regularly held event around the world
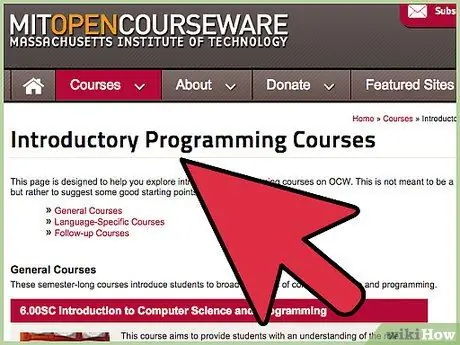
Step 3. Take a programming class
You don't need to study Informatics Engineering, but taking programming classes will really help your learning process. There is no greater help than the help of someone who is familiar with a programming language inside and out. You can take programming classes at youth centers and nearby colleges, and some colleges allow you to take their classes without being a student.
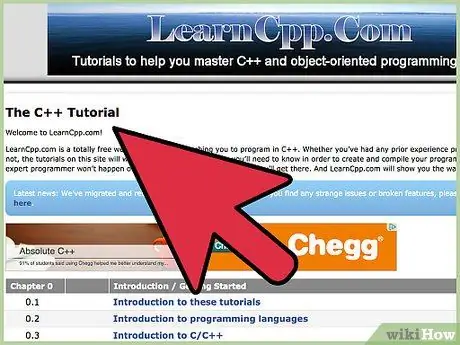
Step 4. Learn C++
Once you understand C, it never hurts to learn C++. C++ is a modern version of C which is more flexible. C++ was designed with object handling in mind, and understanding C++ will allow you to create powerful programs for a variety of operating systems.
Tips
- Always add comments to your program. Comments not only help other people see your code, they also help you remember what you wrote, and why you wrote the code. You may know what you wrote right now, but after two or three months, you won't remember it.
- Always end statements like printf(), scanf(), getch() etc with a semicolon, but don't use semicolons in loop control statements like "if", "while" or "for".
- When experiencing syntax errors in compilation, do a Google search if you are confused. Most likely someone else has experienced the same thing and posted a solution.
- Your C source code should have a *. C extension, so the compiler can understand that your file is C source code.
- Remember that diligent is always clever. The more diligently you practice programming, the faster you will be able to program smoothly. Start with short, simple programs until you're fluent, and once you're confident, you can work on more complex programs.
- Try to learn the logic structure as it will be very helpful when writing code.