DLL files are dynamic linked library files written and controlled through the C++ programming language. DLLs simplify the process of sharing and storing code. This wikiHow teaches you how to create a DLL file using Visual Studio, Windows applications, or Visual Studio for Mac. Make sure you check the “Desktop Development with C++” option in the program installation process. If you already have a Visual Studio program, but don't check the box for that option, you'll need to run the installation file again to make sure the box can be checked.
Step
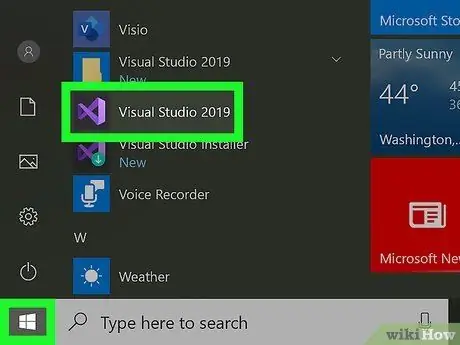
Step 1. Open Visual Studio
You can find this program in the "Start" menu or in the "Applications" folder. Because the DLL file is an information library, it is a "chunk" of the project and usually requires a companion application to be accessed.
- You can download Visual Studio for Windows at this site:
- Visual Studio for Mac can be downloaded here:
- This wikiHow uses code provided by Microsoft to explain how to create a DLL file.
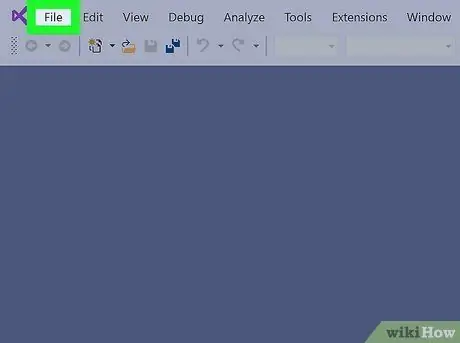
Step 2. Click File
This tab is at the top of the project area (Windows) or at the top of the screen (Mac).
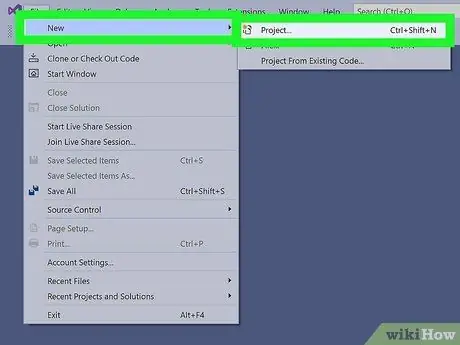
Step 3. Click New and Projects.
The “Create a New Project” dialog box will be displayed.
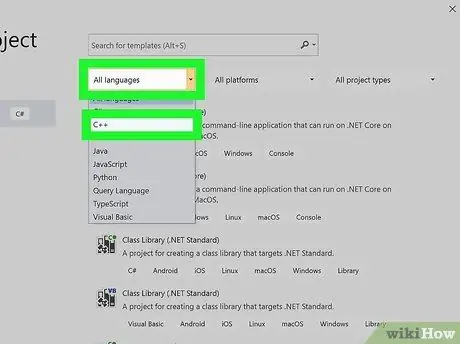
Step 4. Specify the options for the “Language”, “Platform”, and “Project Type” aspects
These aspects will filter the project templates that are displayed.
Click " Language ” to display the drop-down menu and click “ C++ ”.
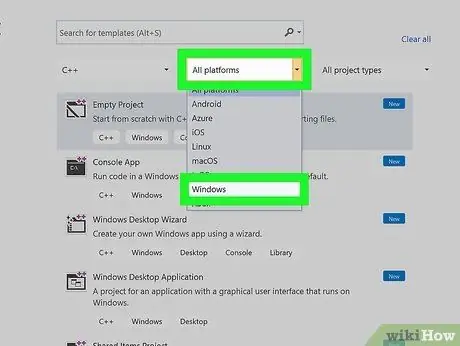
Step 5. Click “Platforms ” to display the drop-down menu and click “ Windows”.
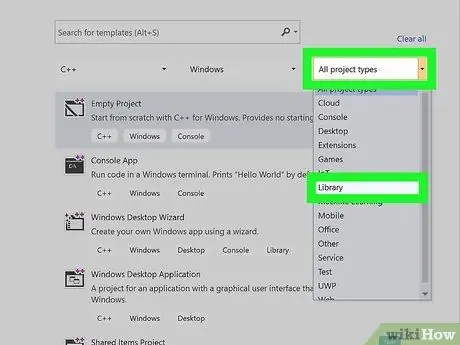
Step 6. Click “Project Type ” to display the drop-down menu and select “ Libraries.
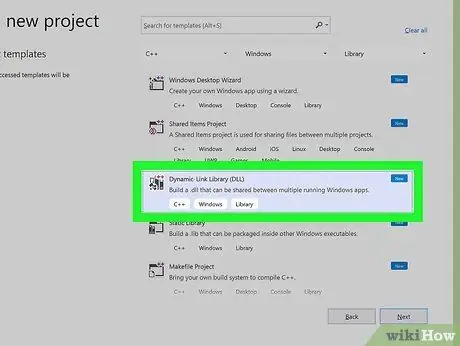
Step 7. Click Dynamic-link Library (DLL)
Options will be marked in blue. Click " Next " to continue.
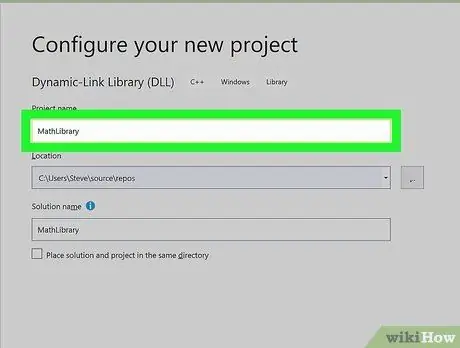
Step 8. Type the project name in the “Name Box” field
For example, you can type “MathLibrary” in the column as an example name.
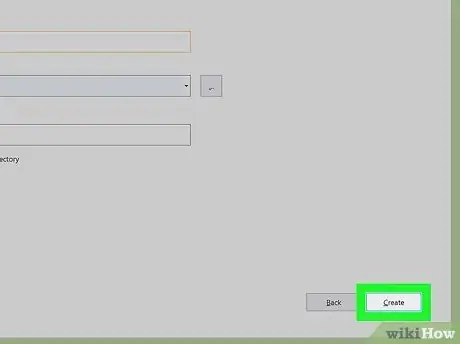
Step 9. Click Create
A DLL project will be created.
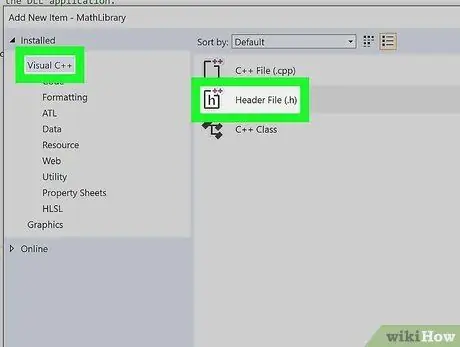
Step 10. Add a header file to the DLL project
You can add it by clicking “Add New Item” from “Project” on the menu bar.
- Choose " Visual C++ ” from the menu on the left side of the dialog box.
- Choose " File headers (.h) ” from the middle of the dialog box.
- Type a name, for example, “MathLibrary.h” into the name field under the menu options.
- Click " Add ” to create an empty header file.
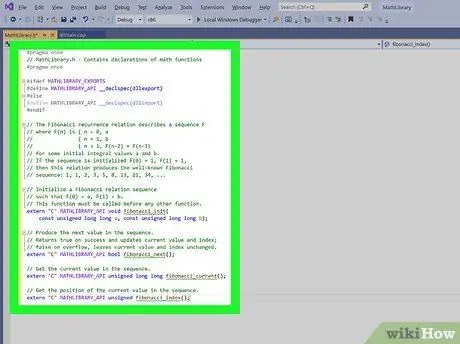
Step 11. Type the following code into the blank header file
// MathLibrary.h - Contains declarations of math functions #pragma once #ifdef MATHLIBRARY_EXPORTS #define MATHLIBRARY_API _declspec(dllexport) #else #define MATHLIBRARY_API _declspec(dllimport) #endif // The Fibonacci recurrence relation F describes a sequence F // where n) is { n = 0, a // { n = 1, b // { n > 1, F(n-2) + F(n-1) // for some initial integral values a and b. // If the sequence is initialized F(0) = 1, F(1) = 1, // then this relation produces the well-known Fibonacci // sequence: 1, 1, 2, 3, 5, 8, 13, 21, 34, … // Initialize a Fibonacci relation sequence // such that F(0) = a, F(1) = b. // This function must be called before any other function. extern "C" MATHLIBRARY_API void fibonacci_init(const unsigned long long a, const unsigned long long b); // Produce the next value in the sequence. // Returns true on success and updates current value and index; // false on overflow, leaves current value and index unchanged. extern "C" MATHLIBRARY_API bool fibonacci_next(); // Get the current value in the sequence. extern "C" MATHLIBRARY_API unsigned long long fibonacci_current(); // Get the position of the current value in the sequence. extern "C" MATHLIBRARY_API unsigned fibonacci_index();
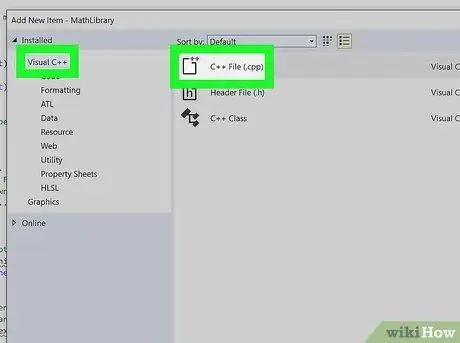
Step 12. Add the CPP file to the DLL project
You can add it by clicking “Add New Item” from “Project” on the menu bar.
- Select “Visual C++” from the menu on the left side of the dialog box.
- Select “C++ File (.cpp)” from the center of the dialog box.
- Type the name “MathLibrary.cpp” into the name field under the menu options.
- Click “Add” to create an empty file.
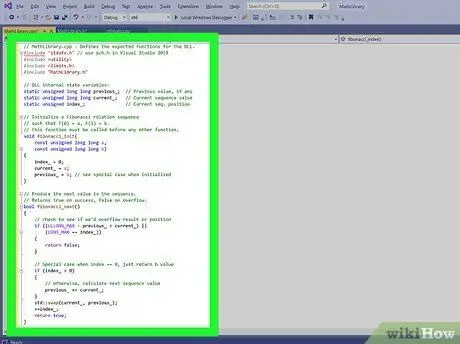
Step 13. Type the following code into the blank file
// MathLibrary.cpp: Defines the exported functions for the DLL. #include "stdafx.h" // use pch.h in Visual Studio 2019 #include #include #include "MathLibrary.h" // DLL internal state variables: static unsigned long long previous_; // Previous value, if any static unsigned long long current_; // Current sequence value static unsigned index_; // Current seq. position // Initialize a Fibonacci relation sequence // such that F(0) = a, F(1) = b. // This function must be called before any other function. void fibonacci_init(const unsigned long long a, const unsigned long long b) { index_ = 0; current_ = a; previous_ = b; // see special case when initialized } // Produce the next value in the sequence. // Returns true on success, false on overflow. bool fibonacci_next() { // check to see if we'd overflow result or position if ((ULLONG_MAX - previous_ < current_) || (UINT_MAX == index_)) { return false; } // Special case when index == 0, just return b value if (index_ > 0) { // otherwise, calculate next sequence value previous_ += current_; } std::swap(current_, previous_); ++index_; return true; } // Get the current value in the sequence. unsigned long long fibonacci_current() { return current_; } // Get the current index position in the sequence. unsigned fibonacci_index() { return index_; }
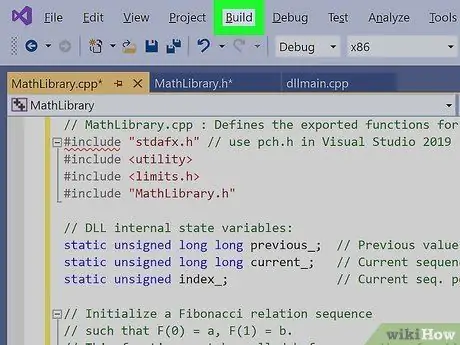
Step 14. Click Build on the menu bar
This option is at the top of the project area (Windows) or at the top of the screen (Mac).
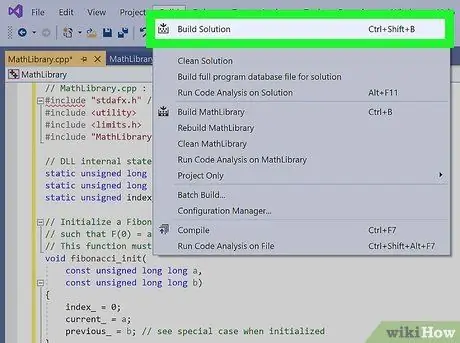
Step 15. Click Build Solution
Once the option is clicked, you can see text like this:
1>------ Build started: Project: MathLibrary, Configuration: Debug Win32 ------ 1>MathLibrary.cpp 1>dllmain.cpp 1>Generating Code… 1> Creating library C:\Users\username \Source\Repos\MathLibrary\Debug\MathLibrary.lib and object C:\Users\username\Source\Repos\MathLibrary\Debug\MathLibrary.exp 1>MathLibrary.vcxproj -> C:\Users\username\Source\Repos\ MathLibrary\Debug\MathLibrary.dll 1>MathLibrary.vcxproj -> C:\Users\username\Source\Repos\MathLibrary\Debug\MathLibrary.pdb (Partial PDB) ========== Build: 1 succeeded, 0 failed, 0 up-to-date, 0 skipped ==========