Variables are one of the most important concepts in computer programming. Variables store information such as letters, numbers, words, sentences, true/false, and more. This article is an introduction to how to use variables in Java. This article is not intended as a complete guide, but as a stepping stone into the world of computer programming.
Step
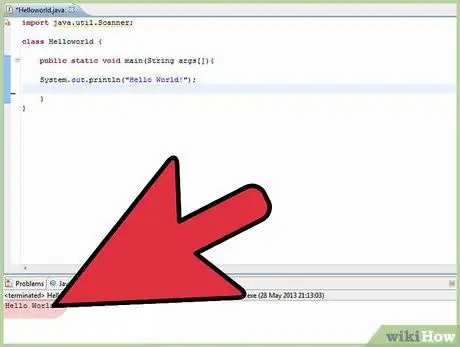
Step 1. Create a simple Java program
The example provided here is named Halo.java:
public class Hello { public static void main(String args) { System.out.println("Hello World!");
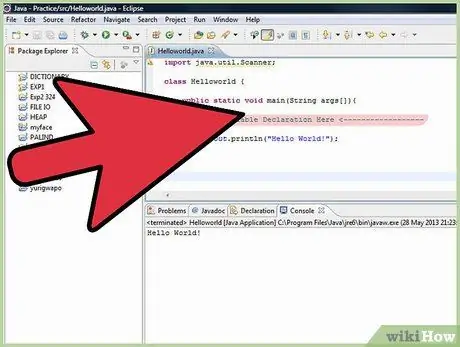
Step 2. Scroll to where you want to insert the variable
Remember: if you place a variable in the main class, you can refer to it anywhere. Select the type of variable you need.
-
Integer data type: used to store integer values like 3, 4, -34 etc.
- bytes
- short
- int
- long
-
Floating Point data type: used to store numbers that contain fractional parts such as 3, 479
- float
- double
-
Character data type (Character): used to store characters like 's', 'r', 'g', 'f' etc.
char
-
Boolean data type: can store one of two values: true and false
boolean
-
Reference data type (Reference): used to store references to objects
- Array Type
- Object Types like String
-
Create a variable. The following is an example of how to create and define values for each type.
318448 3 -
int someNumber = 0;
318448 3b1 -
double someDouble = 635.29;
318448 3b2 -
float someDecimal = 4.43f;
318448 3b3 -
boolean trueFalse = true;
318448 3b4 -
String someSentence = "My dog ate a toy";
318448 3b5 -
char someChar = 'f';
318448 3b6
-
-
Know how it works. Basically, the trick is "type name = value".
318448 4 1 -
Protect the variable from being edited again, optionally, by adding "final type name" between the brackets on the second line of your code (public static void main).
318448 5 1 final int someNumber = 35; Adding 'final' here means that the variable 'someNumber' is immutable
Tips
- Each variable in a program must have a unique name or you will run into errors.
- In Java, all command lines must end with;
- Different variables can have the same name under certain conditions. For example, a variable in a method can have the same name as the example variable name.