There are many ways to program a computer. Ultimately, the decision on how to achieve what is needed rests with the programmer. However, there are many "best practices" of using styles and functions for better compilation and programs. It takes a bit of precision to make sure the next programmers (including yourself) in the project can read and understand your code.
Step
Method 1 of 2: Writing Standard Code
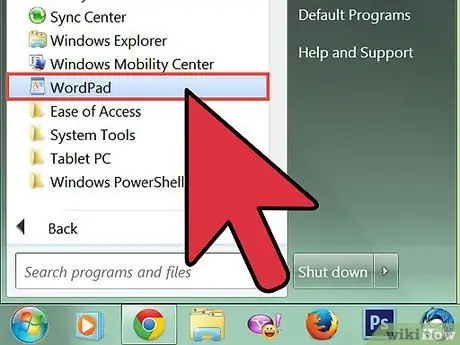
Step 1. Download an IDE (integrated development environment) for C++ such as Eclipse, Netbeans, and CodeBlocks, or you can use a plain text editor such as Notepad++ or VIM
You can also run the program from the command line, in which case any text editor will suffice. It might be useful if you choose an editor that supports syntax highlighting and line numbering. Most programmers find that Unix-like systems (Linux, OS X, BSD) are the best environments for development.
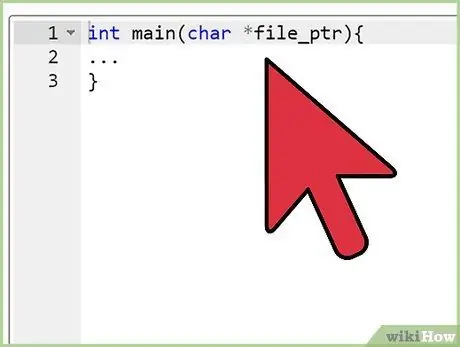
Step 2. Create the main program file
The main file must include a function named main(). This is where program execution begins. From here, you'll need to call functions, prefix classes, etc. Other files from your application as well as libraries can be included in this file.
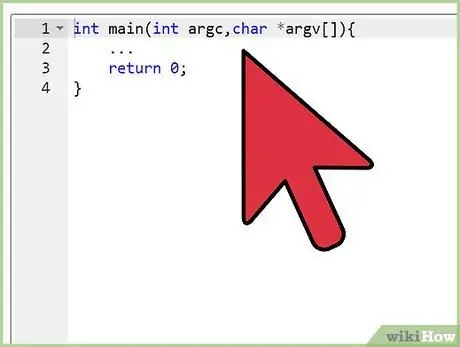
Step 3. Start writing the program
Enter the code or program you want to create (see some examples below). Learn syntax, semantics, Object Oriented Programming paradigm, data striation, design of algorithms like linked lists, priority queues, etc. C++ is not an easy language to program, but doing so will teach you the basics that can be used in all programming languages.
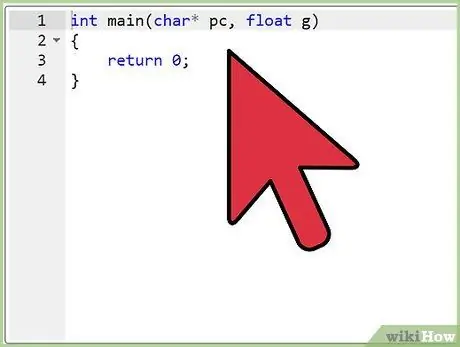
Step 4. Enter the comments in the code
Explain what functions and variables are used for. Choose clear names for variables and functions. Take advantage of global variable names. In general, make sure that anyone reading your code can understand it.
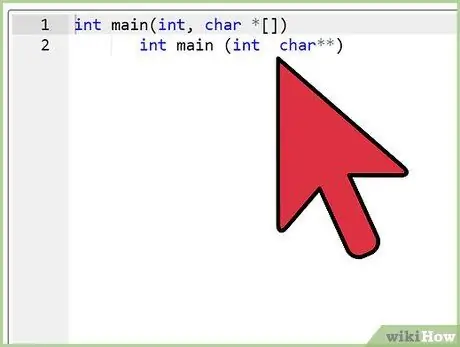
Step 5. Use appropriate indents in your code
Again, see the example below.
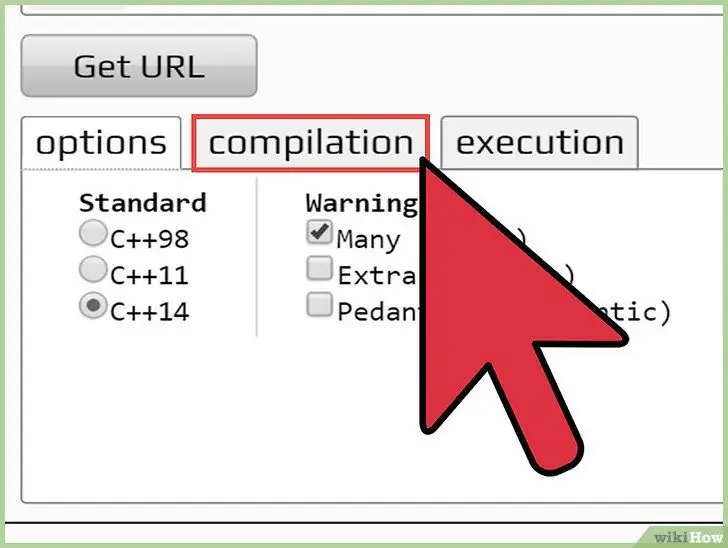
Step 6. Compile the code with
g++ main.cpp
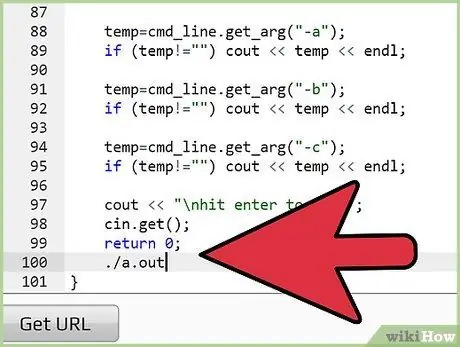
Step 7. Run the program by typing:
./a.out
Method 2 of 2: Example
Step 1. Consider Example 1:
/* This Simple Program is Made to Understand the Basics of g++ Style. This Program Uses the g++ Compiler.*/ #include /* enter input and output functions */ using namespace std; /* we use the std (standard) function */ int main() /* declare the main function; You can also use int main(void). */ { cout << "\n Hello Dad"; /* '\n' is a new line (t is a new tab) */ cout << "\n Hello Mom"; cout << "\n This is my first program"; cout << "\n Date 2018-04-20"; returns 0; }
Step 2. Consider Example 2:
/* This Program To Count The Sum Of Two Numbers */ #include using namespace std; int main() { float num1, num2, res; /* declare variable; int, double, long… can also be used */ cout << "\n Enter first number = "; cin >> num1; /* enter user value into num1 */ cout << "\n Enter second number= "; cin >> num2; res = num1 + num2; cout << "\n Sum "<< num1 <<" and "<< num2 <<" = "<<res '\n'; returns 0; }
Step 3. Study Example 3:
/* Multiply Two Numbers */ #include using namespace std; int main() { float num1; int num2; double res; cout << "\n Enter the first number = "; cin >> num1; cout << "\n Enter the second number = "; cin >> num2; res = num1 * num2; cout << "\n Multiply two numbers = " << res '\n'; returns 0; }
Step 4. Consider Example 4:
// ''Looping'' to find the math formula. In this case, the program finds out the answer to // Question #1 in Project Euler. #include using namespace std; int main() { // Opening ''Main''. int sum1=0; int sum2=0; int sum3=0; int sum4=0; // Generate the integer needed to find the answer. for (int a=0; a < 1000; a=a+3) {sum1 = sum1+a;} // ''Loop'' until a is greater than or equal to 1000, adding 3 each ''loop''. Also add a to sum1. for (int b=0; b < 1000; b=b+5) {sum2 = sum2+b;} // ''Loop'' until b is greater than or equal to 1000, adding 5 each ''loop''. Also add b to sum2. for (int c=0; c < 1000; c=c+15) {sum3 = sum3+c;} // ''Loop'' until c is greater than or equal to 1000, adding 15 to c every ''loop'' '. Also add c to sum3. sum4 = sum1 + sum2 - sum3; // sum4 takes the sum of sum1 and sum2, minus sum3. cout << sum4; // The result is sum4, the answer. cin.get(); // Wait for the user to press Enter. returns 0; // Statement to return. } // Main Closing.
Step 5. Consider the following examples with different styles:
int main(){ int i = 0; if(1+1==2){ i = 2; } } /* Whitesmiths Style */ int main() { int i; if (1+1==2) { i = 2; } } /* GNU Style */ int main() { int i; if (condition) { i = 2; functions(); } }
Tips
- Always use an ISO compiler for your programs.
- 'a.out' is the default executable filename generated by the compiler.
- If you're writing anything that uses a lot of different variables or functions, include some comments to make it easier to debug and understand later!