Changing the colors and text in a C program can help it stand out when run by the user. Changing the color of text and objects is a fairly straightforward process, and the necessary functions are readily available in the standard library. You can change whatever color you produce on the screen.
Step
Part 1 of 2: Changing the Output Text Color
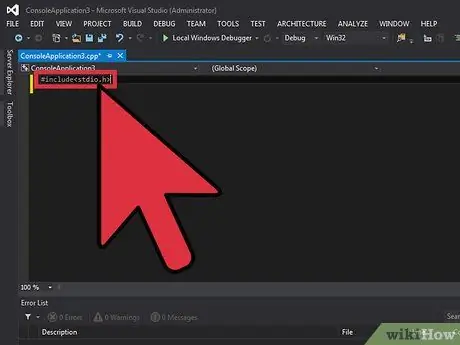
Step 1. Include the Standard Input and Output library
This general library allows you to change the color that the output text displays. Add the following code above your program:
#include
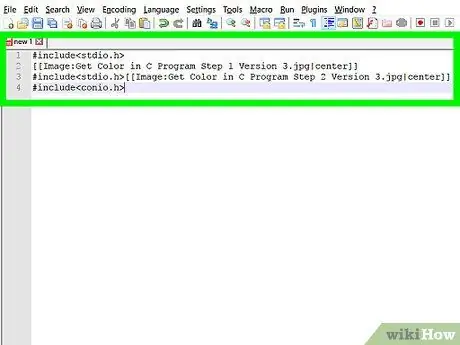
Step 2. Include the Console Input and Output libraries
This step will make it easier to capture keyboard input from users. Add those libraries under the stdio.h library:
#include #include
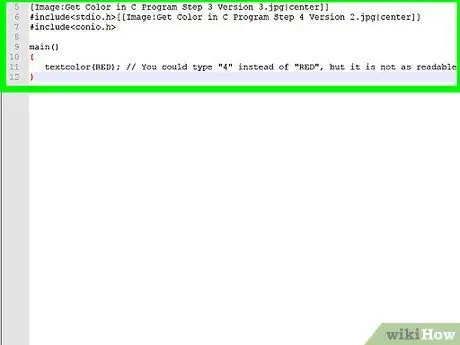
Step 3. Use the textcolor function to specify what color you want to use for the text
You can use this function to vary the color of your output text. Colors must be written in all caps, or expressed as a numeric value:
#include #include main() { textcolor(RED); // You can type "4" instead of "RED", but it's harder to spot }
Color | Numerical Value |
---|---|
BLACK | 0 |
BLUE | 1 |
GREEN | 2 |
SIAN | 3 |
RED | 4 |
MAGENTA | 5 |
CHOCOLATE | 6 |
LIGHT GRAY | 7 |
OLD GRAY | 8 |
LIGHT BLUE | 9 |
LIGHT GREEN | 10 |
SIAN YOUNG | 11 |
PINK | 12 |
YOUNG MAGENTA | 13 |
YELLOW | 14 |
WHITE | 15 |
There are many more colors. Available colors depend on the installed graphics driver and current mode. Colors must be written in all capital letters
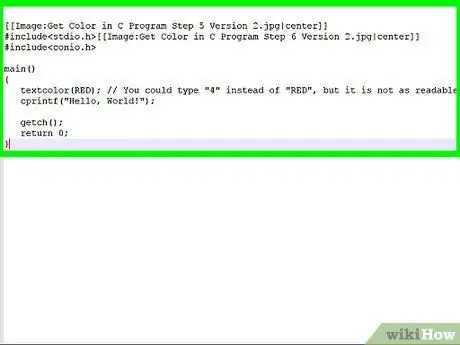
Step 4. Add the output text and finish the program
Include the cprintf function to display some text with your new color. Use the getch function at the end to close the program when the user presses any key.
#include #include main() { textcolor(RED); // You can type "4" instead of "RED", but it's harder to recognize cprintf("Hello, World!"); getch(); returns 0; }
Part 2 of 2: Changing Image Color
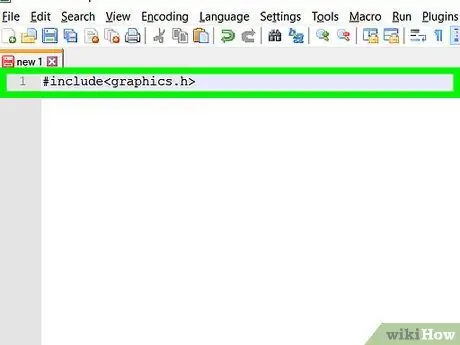
Step 1. Include a graphics library
The C graphics library allows you to draw objects as well as adjust their colors. You can access the graphics library by including it at the top of your program:
#include
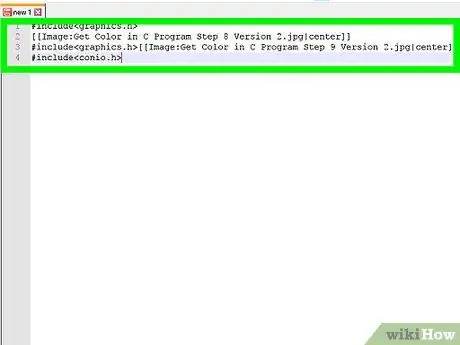
Step 2. Include the Console Input and Output libraries
You can use this library to make it easier to capture user input. Add those libraries under the graphics.h library:
#include #include
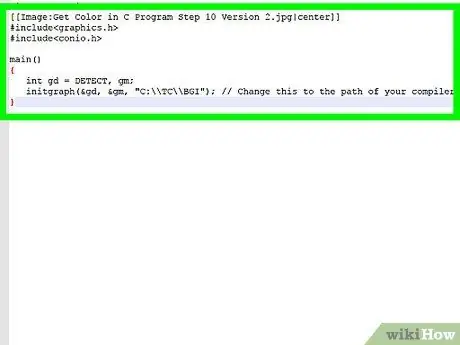
Step 3. Set the variables for the graphics driver and mode
You must perform this step before you start drawing objects so that the program can access the system graphics driver. This step will create an area on the screen where the object is drawn.
#include #include main() { int gd = DETECT, gm; initgraph(&gd, &gm, "C:\TC\BGI"); // Change this to your compiler location }
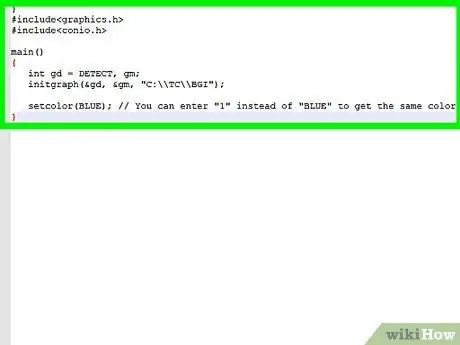
Step 4. Determine the color of the object you want to draw
Before coding an object, use the setcolor function to specify the color of the object to draw:
#include #include main() { int gd = DETECT, gm; initgraph(&gd, &gm, "C:\TC\BGI"); setcolor(BLUE); // You can enter "1" instead of "BLUE" to get the same color, but it's harder to spot }
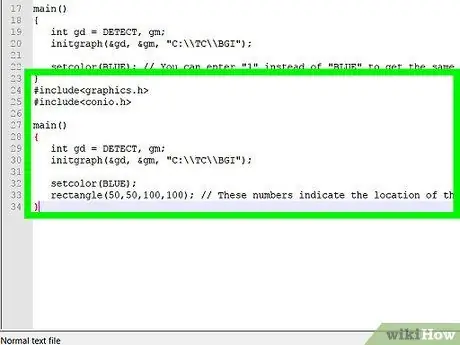
Step 5. Draw the object of your choice
For example, you draw a square using the rectangle function. You can use the graphics.h drawing tool to select a color.
#include #include main() { int gd = DETECT, gm; initgraph(&gd, &gm, "C:\TC\BGI"); setcolor(BLUE); rectangle(50, 50, 100, 100); // This figure shows the location of the top left and bottom right corners }
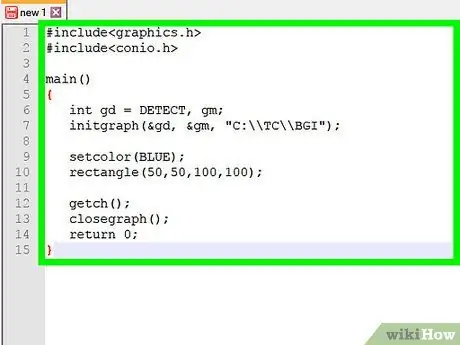
Step 6. Complete the program and run a test run
Add a getch command and turn off the graphics area when you close the program. Do compile and test.
#include #include main() { int gd = DETECT, gm; initgraph(&gd, &gm, "C:\TC\BGI"); setcolor(BLUE); rectangle(50, 50, 100, 100); getch(); closegraph(); returns 0; }
Example
#include #include main() { int gd = DETECT, gm, drawing_color; char a[100]; initgraph(&gd, &gm, ''C:\TC\BGI''); drawing_color = getcolor(); sprintf(a, ''Current drawing color = %d'', drawing_color); outtextxy(10, 10, a); getch(); closegraph(); returns 0; }